Learn about some of the benefits of using GraphQL with Composable Commerce.
After completing this page, you should be able to:
- Identify the advantages GraphQL offers over the REST API.
Some drawbacks to REST APIs in general are overfetching, underfetching, and the use of too many endpoints to find the information you want. For now, let’s just say that both GraphQL and the REST API have their own strengths and weaknesses—and that's why Composable Commerce supports both REST and GraphQL.
REST overfetching
In REST, when you query an endpoint, you get all of the data returned from that endpoint without being able to specify the needed fields. For example, if you use REST to get Customer information, you get the entire Customer record. If, for example, you only want the email addresses of all of your Customers, you waste a lot of bandwidth returning other data fields (first name, last name, address, ID, version, etc.) that you will never use.
With GraphQL, you can specify which elements you want to have returned, so you can query for Customers and only retrieve their email addresses. If that is only 5% of each Customer record, you can retrieve 20 times as many customer records in the same size of data as you can with REST. That’s a big deal.
id
, createdAt
, and totalPrice
fields, alongside the key
of the Customer who placed the Order.Here's the query:
query queryOrdersForCustomers {
orders(where: "createdAt > \"2016-10-01T00:00:00.000Z\"") {
results {
customer {
id
}
id
createdAt
totalPrice {
currencyCode
centAmount
}
}
}
}
Here's what it would look like running through the GraphQL Explorer:
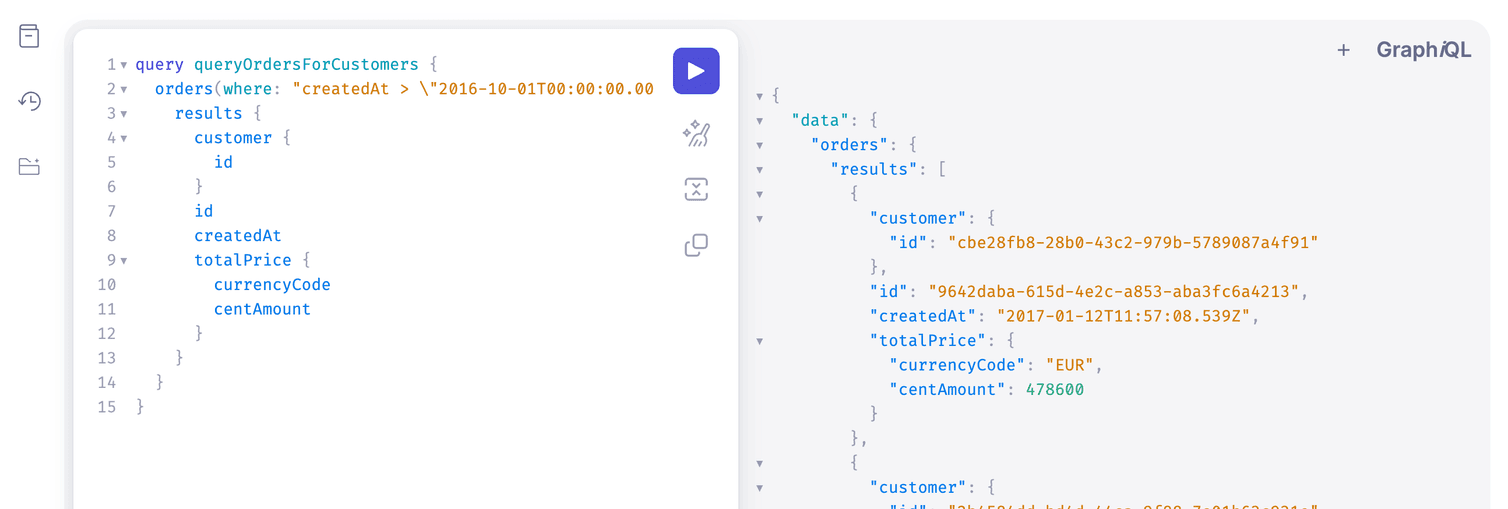
Using the RESTful API for this scenario would lead to a lot of overfetching of unnecessary fields. Here we can see that we only receive the data that we requested. Isn’t it great?
REST underfetching
In REST a related problem to overfetching is underfetching. Again, if you want to read a Customer record with REST, you get all of the information about the Customer, but in Composable Commerce, if a customer belongs to a Customer Group, the only info you get about that is a reference to the Customer Group. Even though you’ve gotten a lot of additional information beyond what you wanted, you still need to send a second request to get the related Customer Group information for that Customer—that’s underfetching.
id
, version
, times for creation, and the last update, as well as information about who did the creation and the last update. Now your underfetching problem is solved—but replaced with even more overfetching.name
field from the referenced Customer Group.Let’s look at another example. Imagine a Product details page and all the data that we want to display on it to our customers. We need some data from the Cart, from Products, some Review rating statistics, and the top 5 Reviews.
Looking at the diagram we can see something that resembles a waterfall request, where each request is made serially. This is not ideal for network latency.
GraphQL solves this problem by allowing us to query data from multiple endpoints in one call.
Rather than multiple requests being made serially, we now have one request only.
To sum it up, GraphQL is a great asset to help you write stable, robust, and performant applications, especially for fetching data.