Learn how to use update actions to update a Customer record with the commercetools SDKs.
After completing this page, you should be able to:
- Identify the correct process for updating a Customer record with the commercetools SDKs.
As we have already seen, we can update a resource with POST request that includes both:
- the correct version number
- an array of update actions
To update a resource in Composable Commerce, you provide the id
or key
to identify the resource to update, and the current version
number with a list of update actions. Versions only exist in the created resource and not the draft payload.
Update actions are a way to explicitly define what changes to make to a resource, without needing to send the whole resource body (including fields that will not change). Here are the Customer update actions:
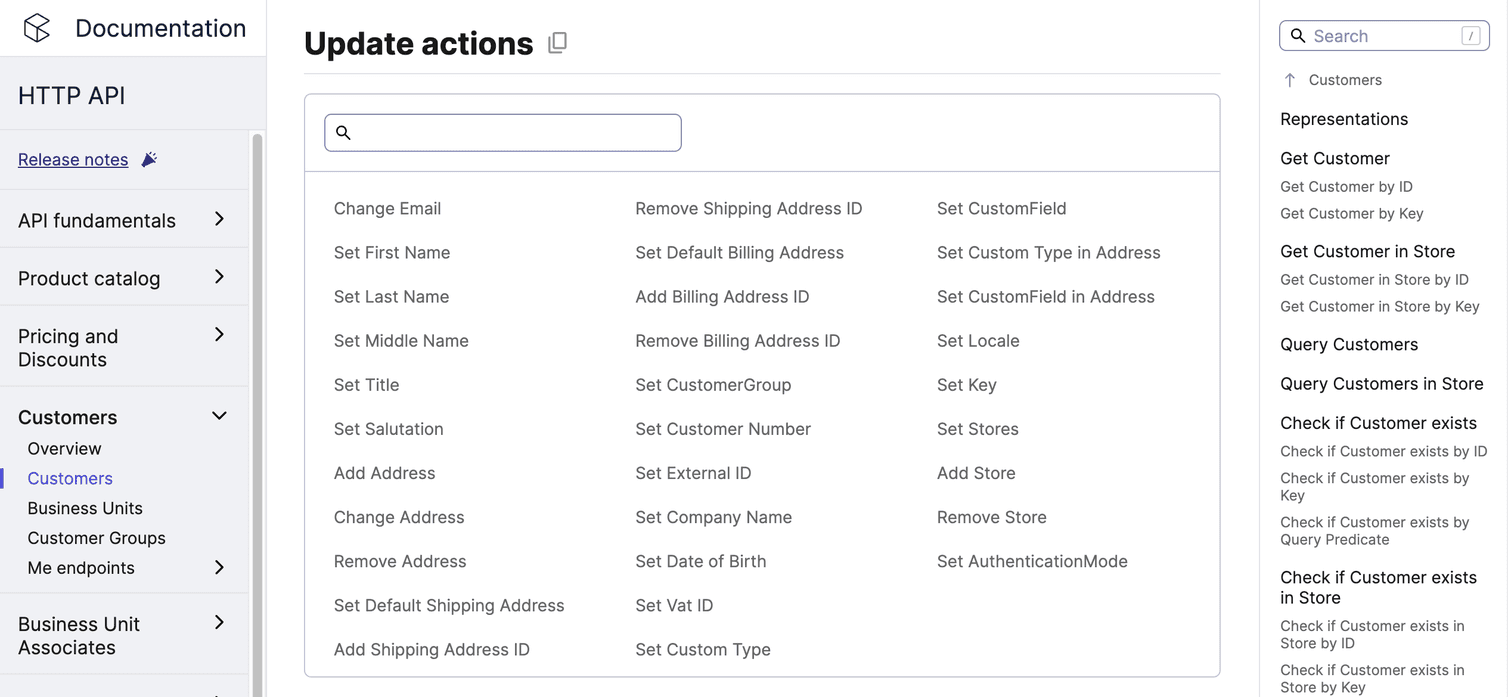
In the following example, we assign the Customer to a Customer Group. We identify the Customer Group with the following key
: vip-customer-group
.
Make sure to create the Customer Group before running the code (you can do this in the Merchant Center).
As with creating a Customer, the Java SDK makes heavy use of the builder pattern. We use this pattern to construct the update action and the reference to the Customer Group. In the TypeScript SDK, you must build the correct structure yourself. However, it is quite interesting how similar both programming languages are in all our exercises.
Make sure you provide the correct version
number in your update actions.
TypeScript SDK
Create a file called customerUpdateGroup.js
and copy the following code:
import { apiRoot } from '../impl/apiClient.js';
const customerKey = 'jon-doe-customer';
const customerGroupId = 'vip-customer-group';
// Change the version number if necessary
const customerVersion = 1;
async function customerUpdateGroup(customerKey, customerGroupId, version) {
try {
const response = await apiRoot
.customers()
.withKey({ key: customerKey })
.post({
body: {
version: version,
actions: [
{
action: 'setCustomerGroup',
customerGroup: { key: customerGroupId },
},
],
},
})
.execute();
console.log('Success', JSON.stringify(response.body, null, 2));
} catch (error) {
console.log(JSON.stringify(error, null, 2));
}
}
customerUpdateGroup(customerKey, customerGroupId, customerVersion);
Java SDK
In the following code we have combined the code snippets from the service and exercise class. Make sure you place the code into the correct files and add import statements as needed.
CustomerService:
public CompletableFuture<ApiHttpResponse<Customer>> assignCustomerToCustomerGroup(long version, String customerKey, String customerGroupKey) {
return
apiRoot
.customers()
.withKey(customer.getKey())
.post(CustomerUpdateBuilder.of()
.version(version())
.actions(
CustomerSetCustomerGroupActionBuilder.of()
.customerGroup(CustomerGroupResourceIdentifierBuilder.of()
.key(customerGroup.getKey())
.build()
)
.build()
)
.build()
)
.execute();
}
CustomerExercise:
logger.info("Customer assigned to group: " +
customerService
.assignCustomerToCustomerGroup(
1L, "jon-doe-customer", "vip-customer-group"
)
.toCompletableFuture().get()
.getBody().getEmail()
);
You might already argue that hardcoding the version number is not a good idea. You are right. We did this to keep the code very short and concise.
Response
Let's first verify that the Customer has been added to the correct Customer Group.
We can check this in the Merchant Center and confirm that John Doe is now a member of vip-customer-group
.
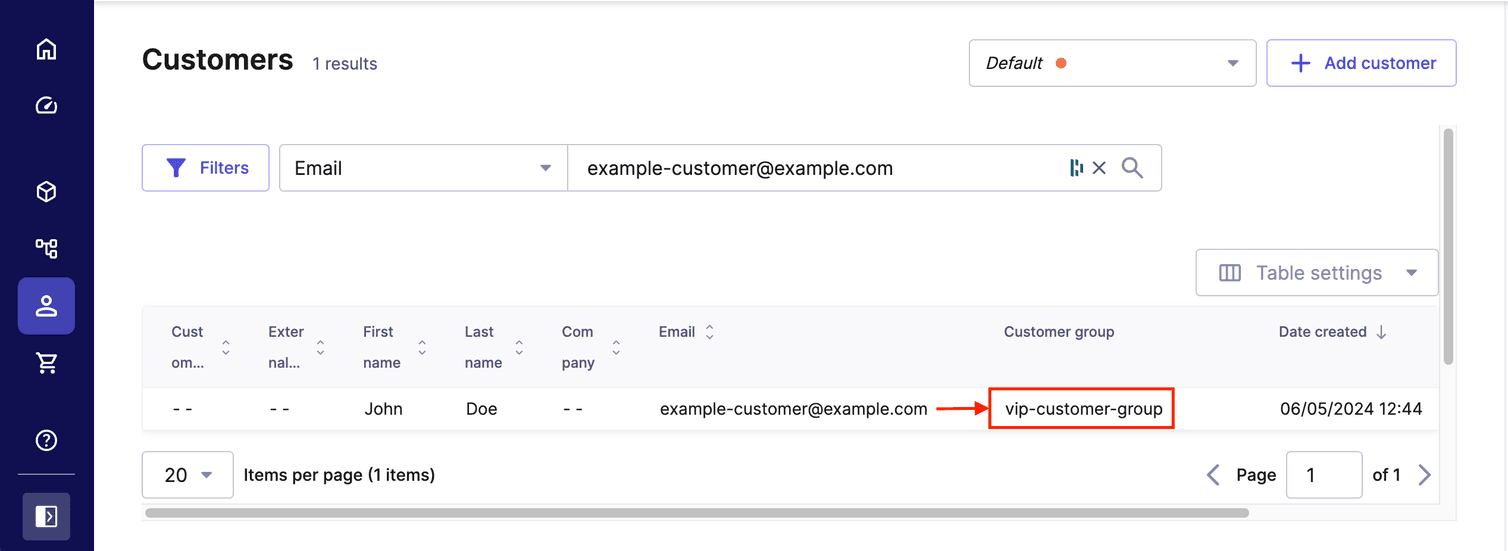
Another example
Let’s look at another example. Here we will add a new address to a Customer's address list. Adding a new address to the Customer's address list uses the addAddress
update action which requires providing information about the address. Check the documentation to understand what fields can be set for this action.
POST https://api.{region}.commercetools.com/{projectKey}/customers/{id}
Authorization: Bearer my_token
Content-Type: application/json
{
"version" : 3,
"actions" : [ {
"action" : "addAddress",
"address" : {
"streetName" : "Easy St",
"streetNumber" : "1",
"postalCode" : "3427",
"city" : "Diggers Rest",
"country" : "AU"
}
} ]
}
Here is how it would look in Postman:
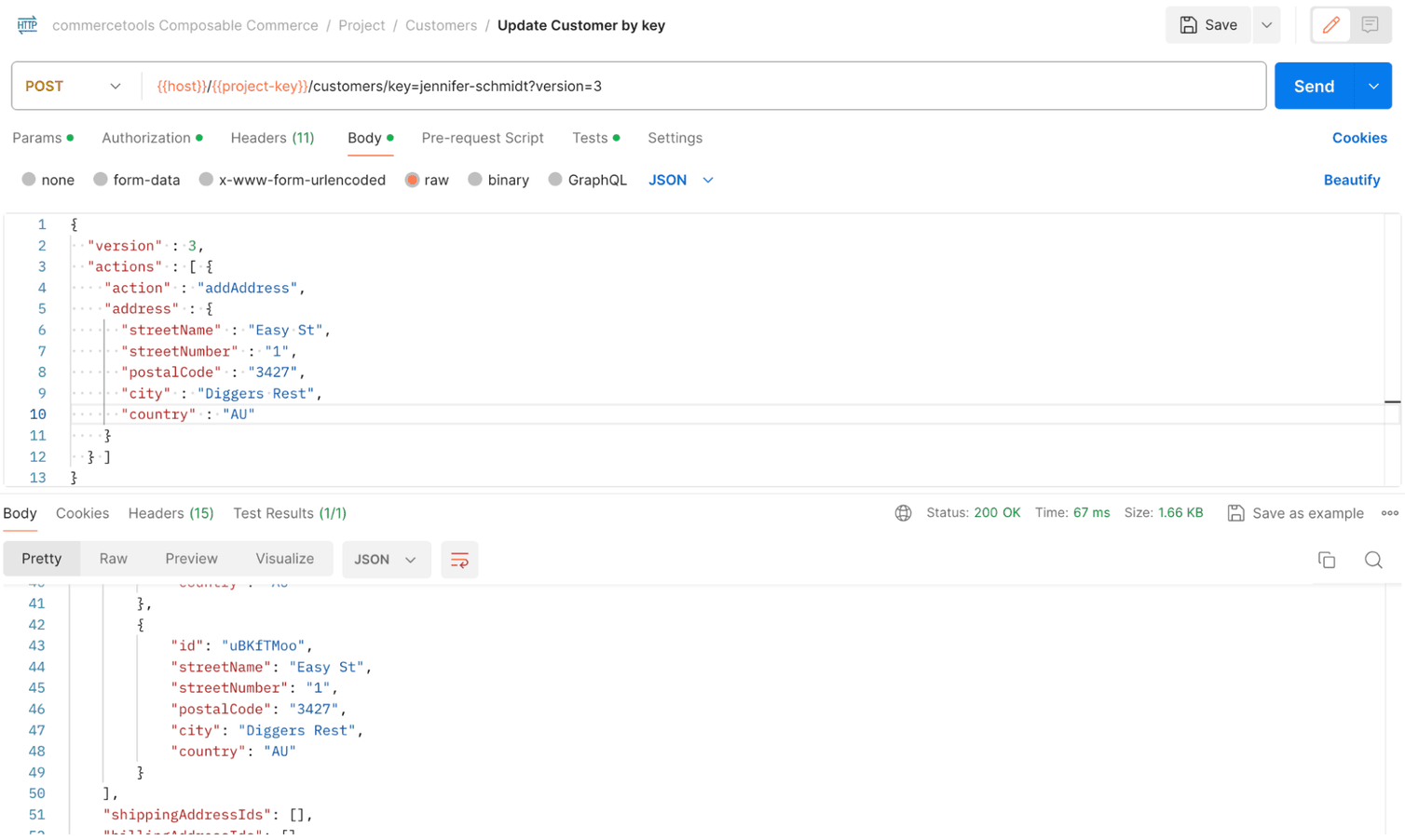
In this example we are adding the address without a key
. If we send multiple addAddress
requests with the correct version and the same address, Composable Commerce will create duplicates of the address, each with a unique id
. This is because we have not set a key
as a unique identifier.
If we set the address including a key
and try to add multiple addresses with the same key
, then we will get a helpful error: The given address with 'key' {key-used} is already present in the Customer.
and no duplicates will be created.
Throughout your Project, using well designed key
s is very important to create and manage resources.
Now check your Customer in your Composable Commerce Project. You will find the new address has been added to the Customer's addresses.