Learn how to configure your environment for the Java SDK.
After completing this module, you should be able to:
- Use the Java SDK to work on a Composable Commerce Project.
The following instructions help you set up your environment to develop applications with Composable Commerce using the Java SDK.
To follow along, you'll need:
- Your preferred Java IDE
- A Java runtime
Pay special attention to version requirements.
Install Java IDE
Install Java runtime environment
Make sure you have a Java environment installed. You can verify it by checking in the terminal with:
java -version
Install the SDK
Let’s create a new local project using IntelliJ by following these steps:
Step 1: Create a new project
Step 2: Name the project
demo
as the project name and saved it in the IdeaProjects
folder.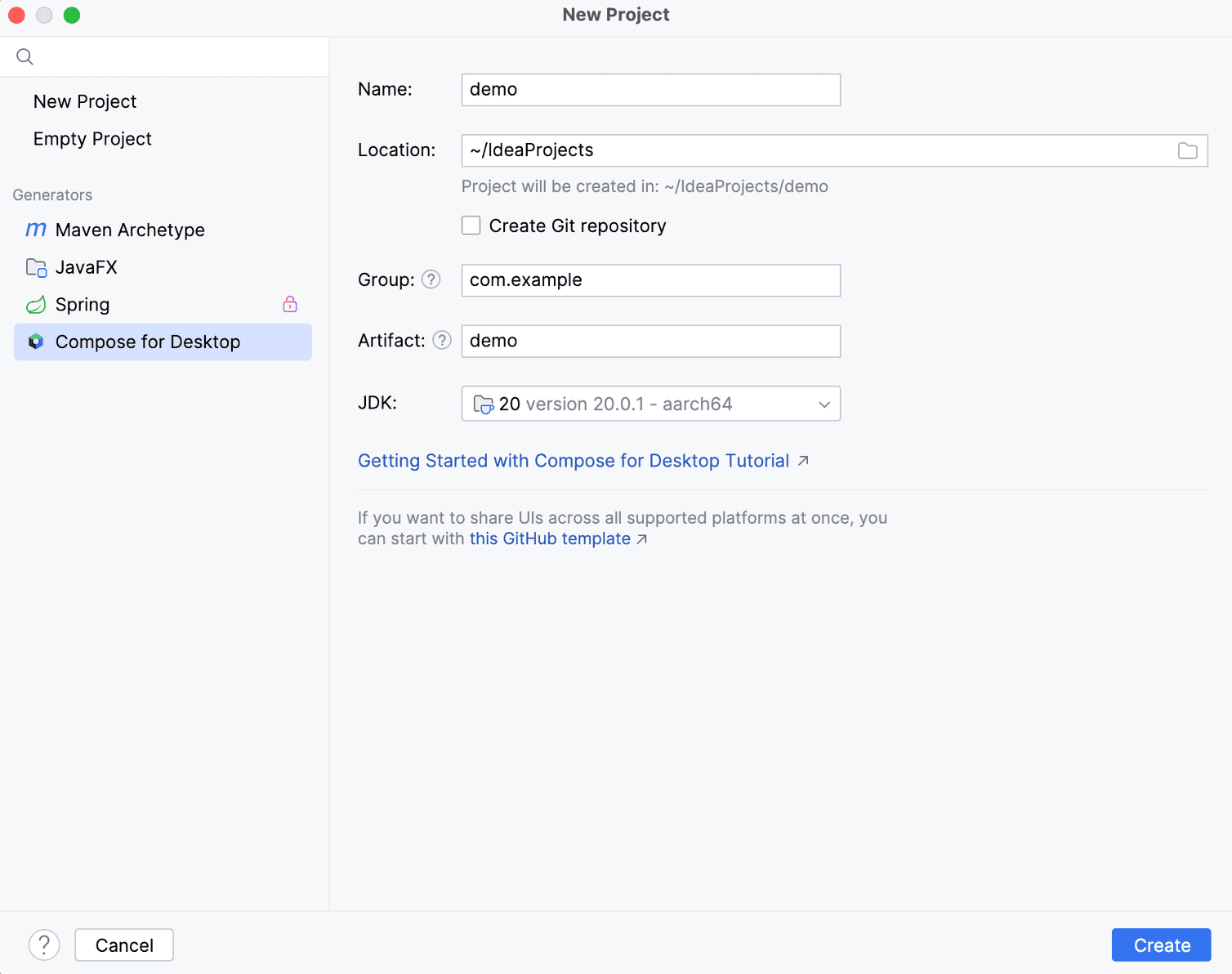
build.gradle
file should look like this:plugins {
id("java")
}
group = "org.example"
version = "1.0-SNAPSHOT"
repositories {
mavenCentral()
}
dependencies {
testImplementation(platform("org.junit:junit-bom:5.7.0"))
testImplementation("org.junit.jupiter:junit-jupiter-engine:5.7.0")
}
tasks.test {
useJUnitPlatform()
}
Step 3: Finish project setup
build.gradle
file.ext {
versions = [
commercetools: "14.5.0",
slf4j: "1.7.36",
logback: "1.2.10",
]
}
allprojects {
apply plugin: 'java'
sourceCompatibility = 1.8
targetCompatibility = 1.8
}
repositories {
mavenCentral()
maven {
url "https://packages.atlassian.com/maven-3rdparty"
}
}
dependencies {
implementation "com.commercetools.sdk:commercetools-http-client:${versions.commercetools}"
implementation "com.commercetools.sdk:commercetools-sdk-java-api:${versions.commercetools}"
implementation "com.commercetools.sdk:commercetools-graphql-api:${versions.commercetools}"
implementation "com.commercetools.sdk:commercetools-sdk-java-importapi:${versions.commercetools}"
implementation "com.commercetools.sdk:commercetools-sdk-java-ml:${versions.commercetools}"
implementation "org.slf4j:slf4j-api:${versions.slf4j}"
implementation "ch.qos.logback:logback-classic:${versions.logback}"
implementation group: 'org.json', name: 'json', version: '20200518'
implementation 'javax.json:javax.json-api:1.1.4'
implementation 'org.glassfish:javax.json:1.1.4'
}
Check that the installation was successful by running the following command in your IDE terminal:
./gradlew build
BUILD SUCCESSFUL
?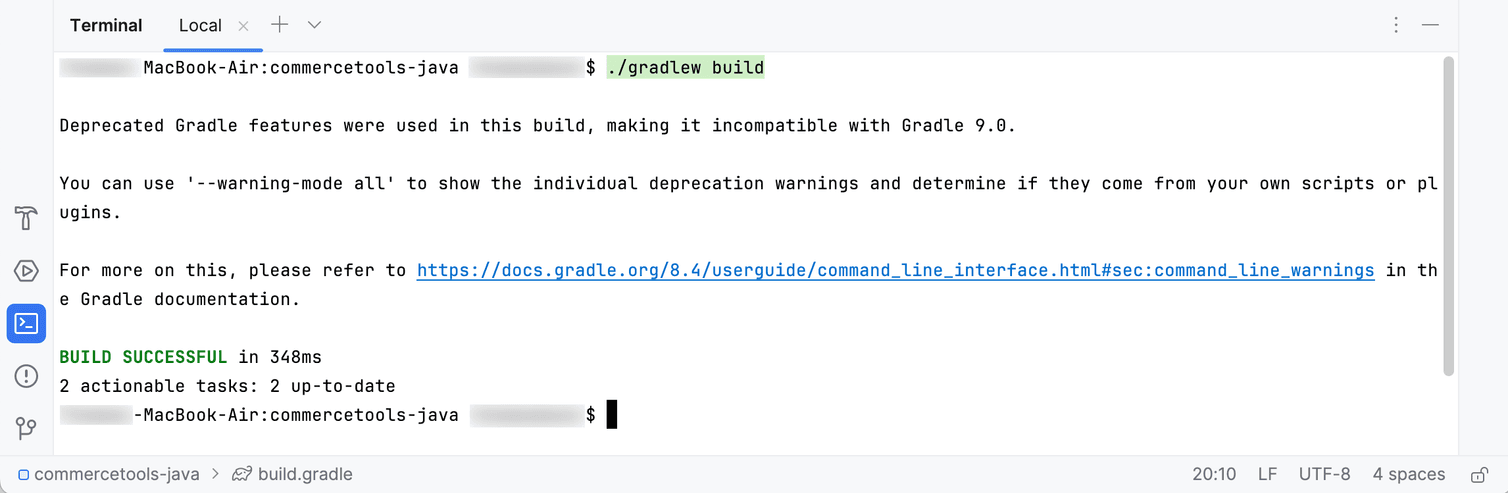
Step 4: Resolve possible errors with the Java SDK
-
Update Gradle wrapper configuration: locate and open the
gradle-wrapper.properties
file within your project directory. Set thedistributionUrl
to https://services.gradle.org/distributions/gradle-6.7-bin.zip. This setting ensures that Gradle 6.7 is used for building the project. -
Check Gradle and Java versions in IntelliJ: navigate to Settings > Build, Execution, Deployment > Build Tools > Gradle. Use the Gradle version specified in the
gradle-wrapper.properties
file. Select Java version 15.0.2 for the Gradle JVM.
Test your setup
Step 5: Set up an API Client in your SDK
ClientService.java
and place it in a folder called /impl
.package impl;
import com.commercetools.api.client.ProjectApiRoot;
import com.commercetools.api.defaultconfig.ApiRootBuilder;
import com.commercetools.api.defaultconfig.ServiceRegion;
import io.vrap.rmf.base.client.oauth2.ClientCredentials;
import java.io.IOException;
public class ClientService {
public static ProjectApiRoot projectApiRoot;
public static ProjectApiRoot createApiClient() throws IOException {
String clientId = "";
String clientSecret = "";
String projectKey = "";
projectApiRoot = ApiRootBuilder.of()
.defaultClient(
ClientCredentials.of()
.withClientId(clientId)
.withClientSecret(clientSecret)
.build(),
ServiceRegion.{region}.getOAuthTokenUrl(),
ServiceRegion.{region}.getApiUrl()
).build(projectKey);
return projectApiRoot;
}
}
Then update the following values to match your API Client:
clientId
clientSecret
projectKey
ServiceRegion.{region}getOAuthTokenUrl()
ServiceRegion.{region}.getApiUrl()
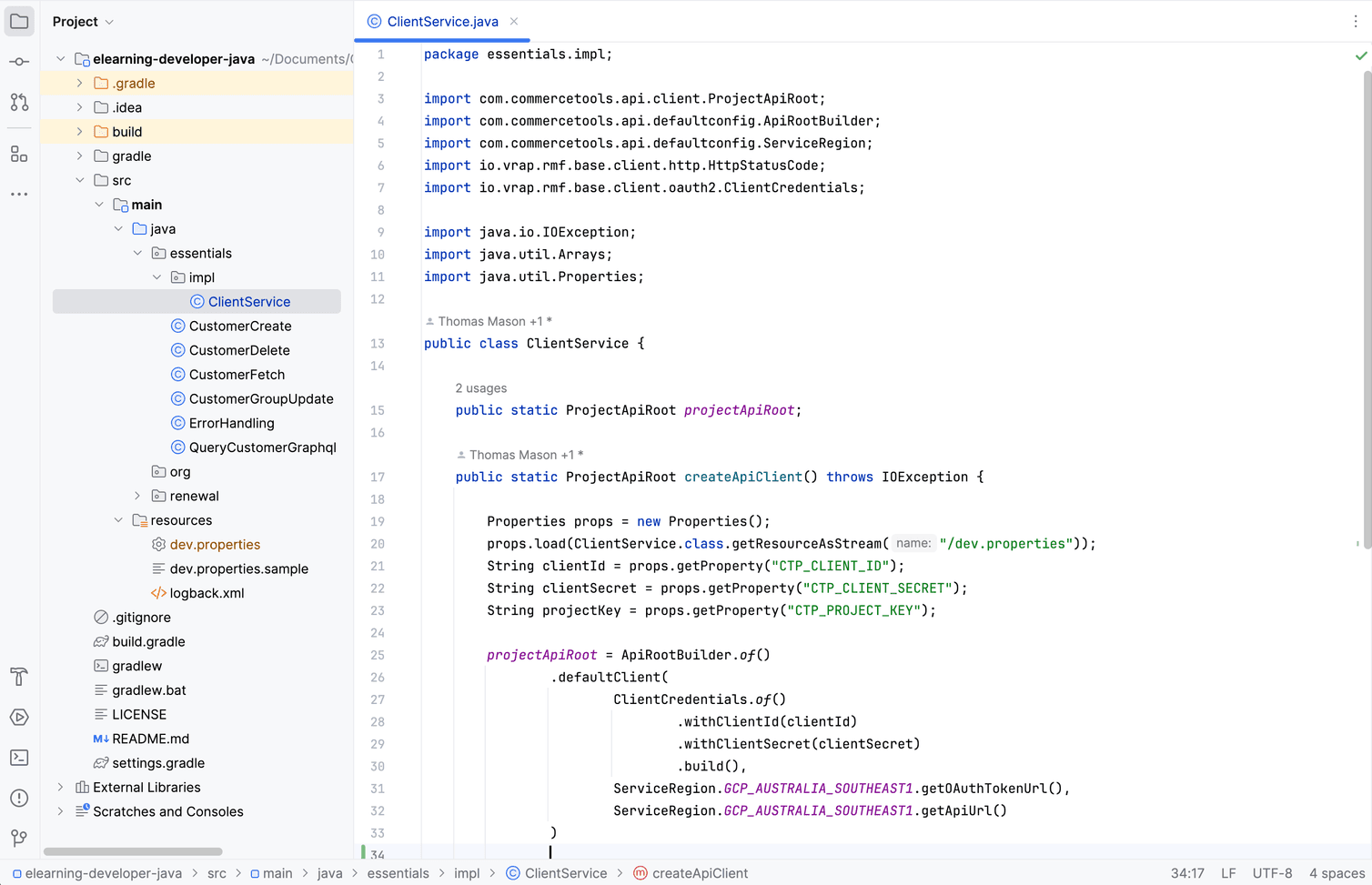
Step 6: Fetch the Customer data
CustomerFetch.java
and copy the following code. Remember to update the Customer ID.import com.commercetools.api.client.ProjectApiRoot;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
import java.io.IOException;
import java.util.concurrent.ExecutionException;
import static impl.ClientService.createApiClient;
public class CustomerFetch {
public static void main(String[] args) throws IOException, ExecutionException, InterruptedException {
final ProjectApiRoot client = createApiClient();
Logger logger = LoggerFactory.getLogger(CustomerFetch.class.getName());
String customerId = "";
logger.info(
"Fetching the customers last name " +
client
.customers()
.withId(customerId)
.get()
.execute()
.toCompletableFuture().get()
.getBody()
.getLastName()
);
client.close();
}
}
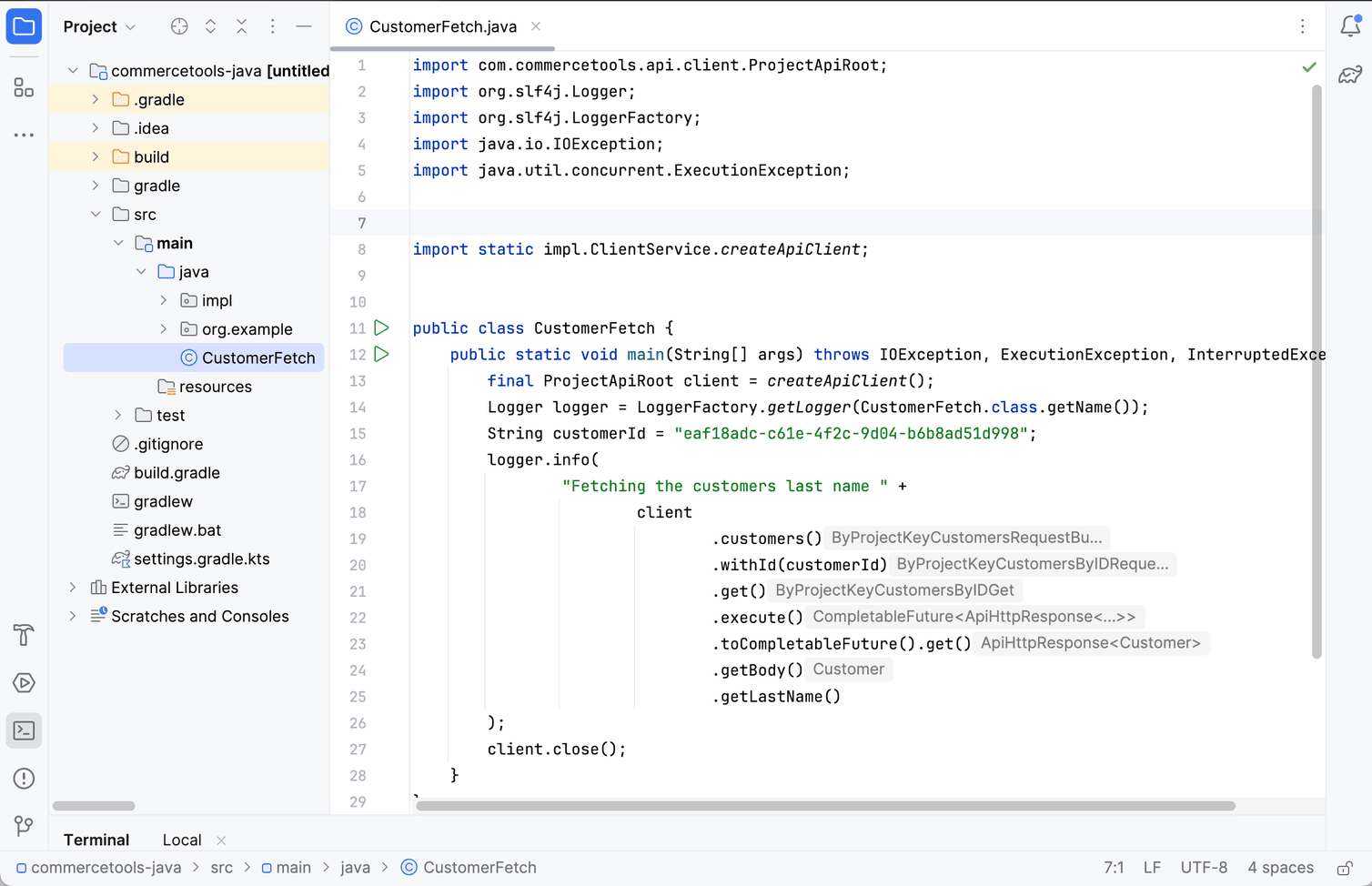
Step 7: Execute the code
You can write your own run task or use the IDE to run the main method.
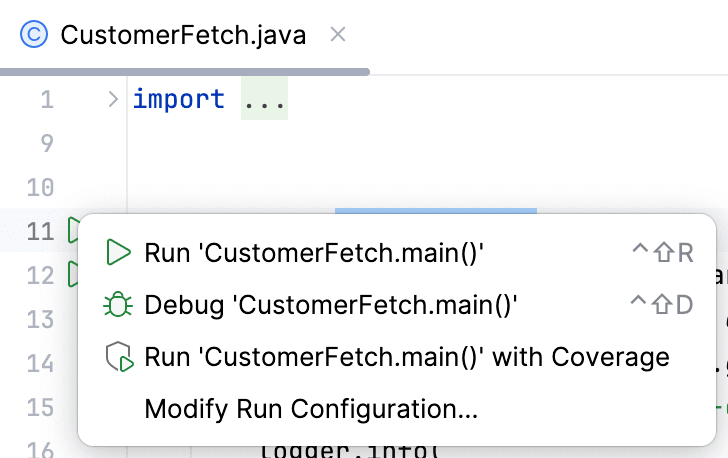
Nice work! Customer successfully fetched!
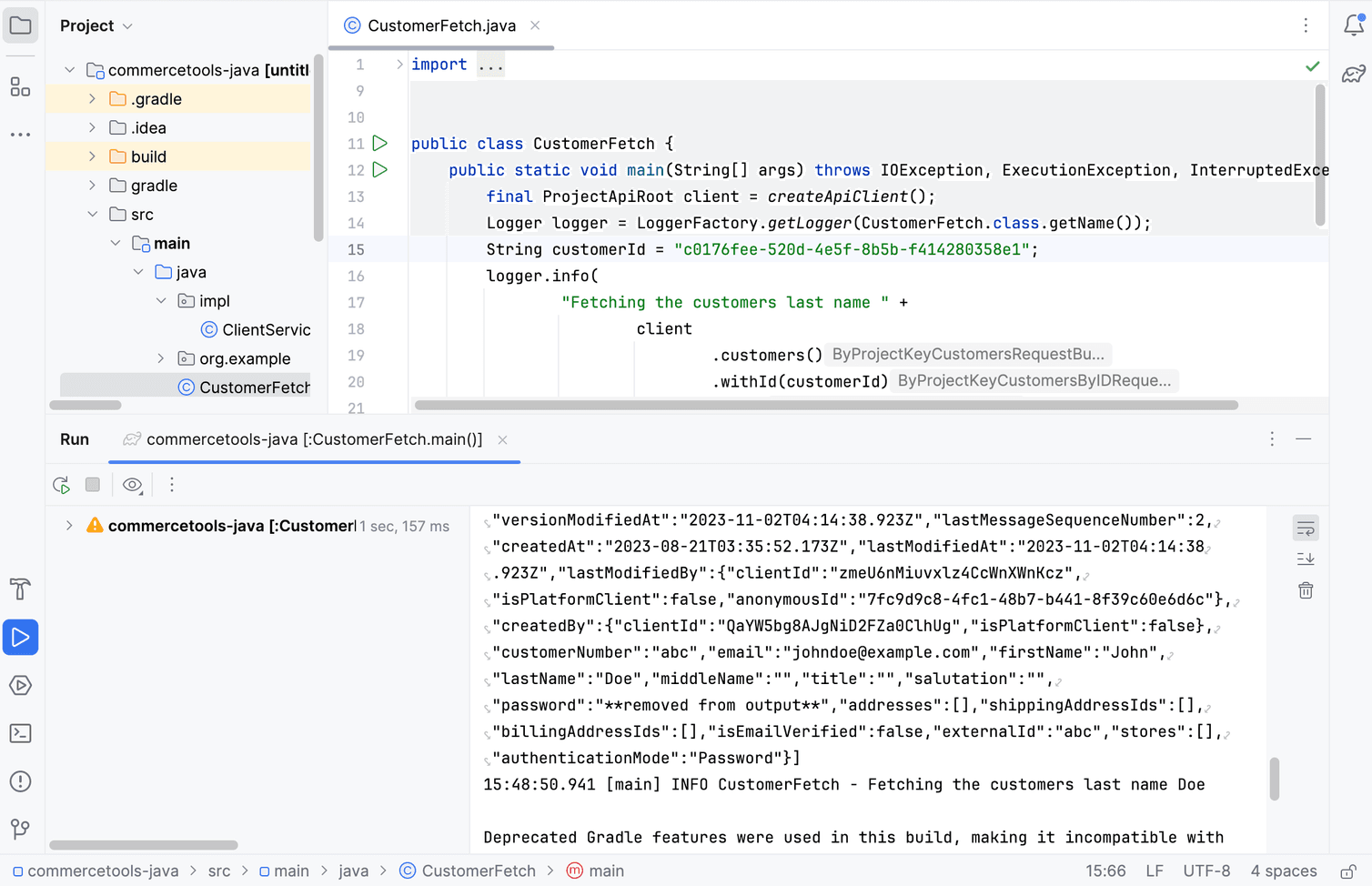