Learn how to set up and use the Java SDK.
This step-by-step guide leads you through setting up and making API calls using the Java SDK.
Requirements
To follow this guide you should have the following:
- A commercetools Composable Commerce Project
- An API Client
- Java 8 (or later)
Objectives of the get started guide
After following this guide you will have:
Placeholder values
Example code in this guide uses the following placeholder values. You should replace these placeholders with the following values.
Placeholder | Replace with | From |
---|---|---|
{projectKey} | project_key | your API Client |
{clientID} | client_id | your API Client |
{clientSecret} | secret | your API Client |
{scope} | scope | your API Client |
{region} | your Region | Hosts |
Install the Java SDK
Gradle
latest.release
with the version number. ext {
versions = [
commercetools: "latest.release"
]
}
repositories {
mavenCentral()
}
dependencies {
implementation "com.commercetools.sdk:commercetools-http-client:${versions.commercetools}"
implementation "com.commercetools.sdk:commercetools-sdk-java-api:${versions.commercetools}"
implementation "com.commercetools.sdk:commercetools-sdk-java-importapi:${versions.commercetools}"
implementation "com.commercetools.sdk:commercetools-sdk-java-history:${versions.commercetools}"
}
Maven
LATEST
with the version number. <properties>
<commercetools.version>LATEST</commercetools.version>
</properties>
<dependencies>
<dependency>
<groupId>com.commercetools.sdk</groupId>
<artifactId>commercetools-http-client</artifactId>
<version>${commercetools.version}</version>
</dependency>
<dependency>
<groupId>com.commercetools.sdk</groupId>
<artifactId>commercetools-sdk-java-api</artifactId>
<version>${commercetools.version}</version>
</dependency>
<dependency>
<groupId>com.commercetools.sdk</groupId>
<artifactId>commercetools-sdk-java-importapi</artifactId>
<version>${commercetools.version}</version>
</dependency>
<dependency>
<groupId>com.commercetools.sdk</groupId>
<artifactId>commercetools-sdk-java-history</artifactId>
<version>${commercetools.version}</version>
</dependency>
</dependencies>
Maven Central
commercetools-http-client
.commercetools-sdk-java-importapi
(Import API) and commercetools-sdk-java-history
(Change History API) are only needed for more specific use cases.Troubleshoot with the Spring Framework
The Spring Framework has an optional dependency to OkHttp in version 3.x.
commercetools-okhttp-client3
or commercetools-apachehttp-client
module instead of the module commercetools-http-client
.Create the Client class
Client
and add the following code:// Add your package information here
// Required imports
import com.commercetools.api.client.ProjectApiRoot;
import com.commercetools.api.defaultconfig.ApiRootBuilder;
import com.commercetools.api.defaultconfig.ServiceRegion;
import io.vrap.rmf.base.client.oauth2.*;
public class Client {
public static ProjectApiRoot createApiClient() {
final ProjectApiRoot apiRoot = ApiRootBuilder.of()
.defaultClient(ClientCredentials.of()
.withClientId("{clientID}")
.withClientSecret("{clientSecret}")
.build(),
ServiceRegion.GCP_EUROPE_WEST1)
.build("{projectKey}");
return apiRoot;
}
}
ServiceRegion.GCP_EUROPE_WEST1
to match the Region where your Project is hosted. For more information about the supported values, see ServiceRegion.Test the Client
In your Java program, add the following code:
// Required imports
import com.commercetools.api.client.ProjectApiRoot;
import com.commercetools.api.models.project.*;
// Create the apiRoot with your Client
ProjectApiRoot apiRoot = Client.createApiClient();
// Make a get call to the Project
Project myProject = apiRoot
.get()
.executeBlocking()
.getBody();
// Output the Project name
System.out.println(myProject.getName());
apiRoot
to build requests to the Composable Commerce API.myProject
object and outputs the Project's name using .getName()
.Use the Java SDK
Imports
Without importing resource-specific packages and interfaces you cannot use/access specific objects and methods.
For example, to use or create a Shopping List you must import:
import com.commercetools.api.models.shopping_list.ShoppingList;
import com.commercetools.api.models.shopping_list.ShoppingListDraft;
com.commercetools.api.models.shopping_list
package using:import com.commercetools.api.models.shopping_list.*;
Use builders
The Java SDK follows a builder pattern when constructing drafts, update actions, and other objects/types that contain multiple fields.
// Create a LocalizedString
LocalizedString multiLanguageString = LocalizedString
.builder()
.addValue("en", "English value")
.addValue("de", "German value")
.build();
// Create US$100.00
Money money = Money.builder().currencyCode("USD").centAmount(10000l).build();
// Create a Category
CategoryDraft categoryDraft = CategoryDraft
.builder()
.name(LocalizedString.ofEnglish("english name"))
.slug(stringBuilder -> stringBuilder.addValue("en", "english-slug"))
.key("category-key")
.build();
.build()
finishes building the object.Structure your API call
Calls to the Java SDK require you to make an instance of the type you want returned, or an instance of an action to take:
// Return the information of a specific Shopping List
ShoppingList shoppingListInfo
// ...
// Return all Shopping Lists
ShoppingListPagedQueryResponse allShoppingLists
// ...
// Create an update action for setting a Shopping List key
ShoppingListSetKeyAction shoppingListSetKeyAction
// ...
Retrieve data
apiRoot
and the associated endpoint.// Get information of a specific Shopping List
ShoppingList shoppingListInfo = apiRoot
.shoppingLists()
// ...
// Return all Shopping Lists
ShoppingListPagedQueryResponse allShoppingLists = apiRoot
.shoppingLists()
// ...
Get a single resource
.get()
, .executeBlocking()
and .getBody();
.// Information of a specific Shopping List by ID
ShoppingList shoppingListInfo = apiRoot
.shoppingLists()
.withId("{shoppingListID}")
.get()
.executeBlocking()
.getBody();
// Information of a specific Shopping List by key
ShoppingList shoppingListInfo = apiRoot
.shoppingLists()
.withKey("{shoppingListKey}")
.get()
.executeBlocking()
.getBody();
shoppingListInfo
object would then contain all the data of the specified Shopping List. You can access information from the fields within that object: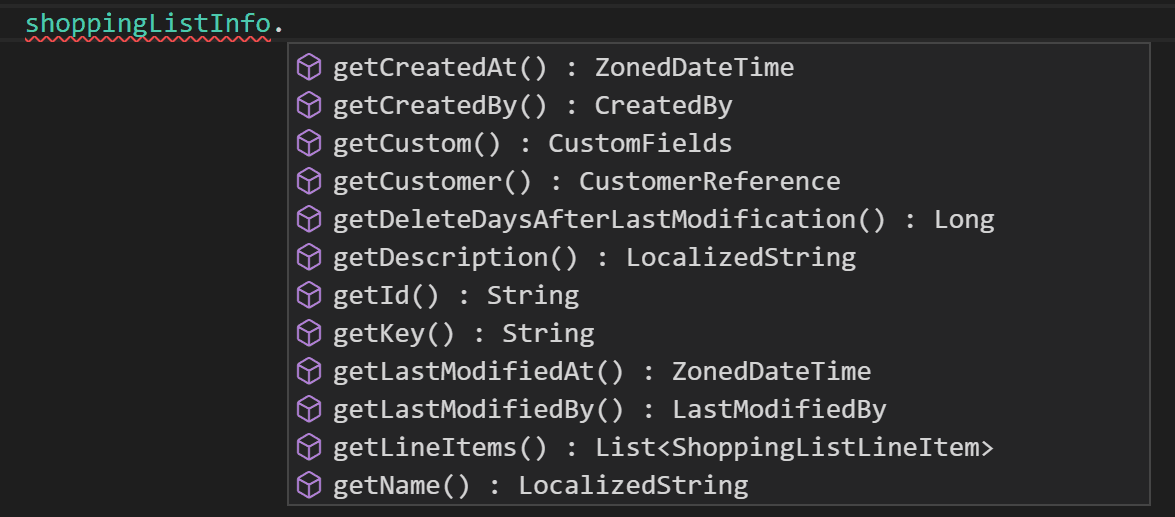
Get multiple resources
PagedQueryResponse
based on the resource you want to return. For example, a ShoppingListPagedQueryResponse
returns Shopping Lists.PagedQueryResponse
is identical to PagedQueryResult in the HTTP API.// Return all Shopping Lists in a PagedQueryResponse
ShoppingListPagedQueryResponse allShoppingLists = apiRoot
.shoppingLists()
.get()
.executeBlocking()
.getBody();
.withWhere()
, .withSort()
, .withExpand()
, .withLimit()
, or .withOffset()
after .get()
.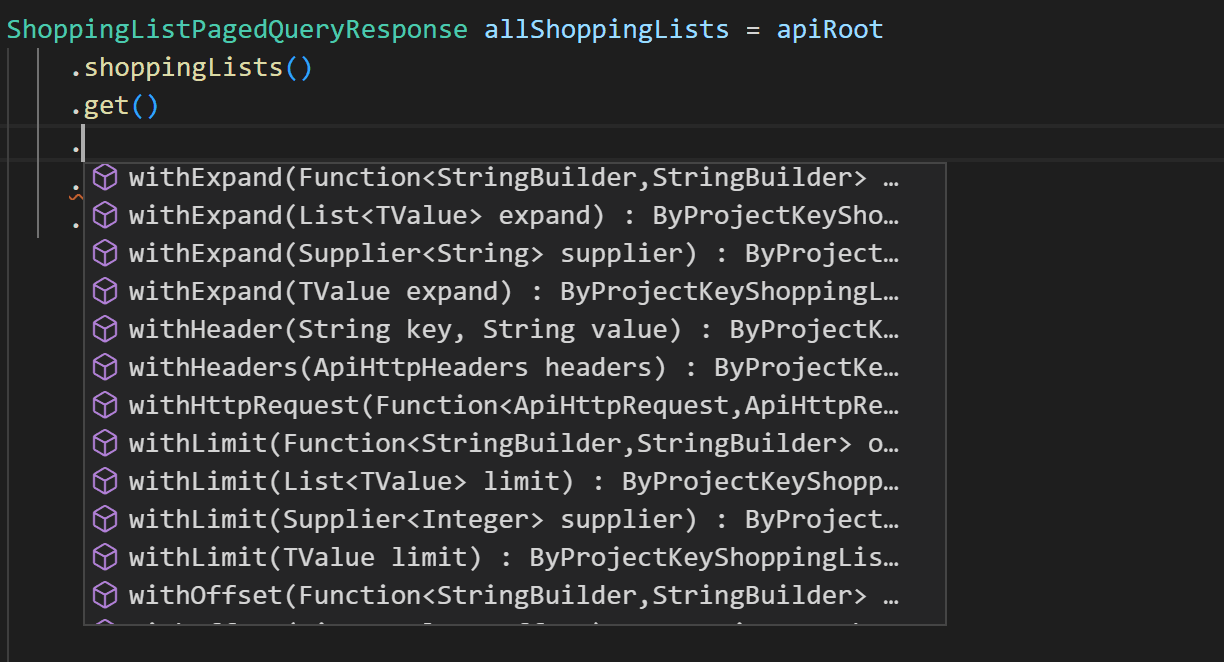
Use the Query Predicate builder
withQuery
method.// Return all Customers that have not verified their email address
final CustomerPagedQueryResponse response = apiRoot
.customers()
.get()
.withQuery(c -> c.isEmailVerified().is(false))
.executeBlocking()
.getBody();
View results
PagedQueryResponse
using .getResults()
:// Return all Shopping Lists
ShoppingListPagedQueryResponse allShoppingLists = apiRoot
.shoppingLists()
.get()
.executeBlocking()
.getBody();
// Put the returned Shopping Lists in a new list
List<ShoppingList> listOfShoppingLists = allShoppingLists.getResults();
// Create a String containing the first Shopping List's English name
String firstShoppingListName = listOfShoppingLists.get(0).getName().get("en");
Write a resource
apiRoot
and the associated endpoint.// Create a Shopping List
ShoppingList newShoppingList = apiRoot
.shoppingLists()
// ...
// Update a Shopping List
ShoppingList updatedShoppingList = apiRoot
.shoppingLists()
// ...
Create a new resource
// Create ShoppingListDraft with required fields
ShoppingListDraft newShoppingListDraft = ShoppingListDraft
.builder()
.name(
LocalizedString
.builder()
.addValue("en", "English name of Shopping List")
.build()
)
.build();
post()
followed by .executeBlocking()
and .getBody()
.// Create a Shopping List
ShoppingList newShoppingList = apiRoot
.shoppingLists()
.post(newShoppingListDraft)
.executeBlocking()
.getBody();
Update an existing resource
.withId()
or .withKey()
that references a unique identifier of the resource.// Update a Shopping List
ShoppingList updatedShoppingList = apiRoot
.shoppingLists()
.withId("{shoppingListID}")
// ...
ShoppingListUpdate
) contains an array of update actions and the last seen version of the resource.// Create the payload - a ShoppingListUpdate - with the current version of the Shopping List and the update actions.
ShoppingListUpdate shoppingListUpdate = ShoppingListUpdateBuilder
.of()
.version(1L)
.plusActions(actionBuilder ->
actionBuilder.setKeyBuilder().key("a-unique-shoppinglist-key")
)
.build();
.post()
method.// Update a Shopping List
ShoppingList updatedShoppingList = apiRoot
.shoppingLists()
.withId("{shoppingListID}")
.post(shoppingListUpdate)
.executeBlocking()
.getBody();
Delete a resource
.delete()
method with the last seen version of the resource. You must identify the resource to delete using withId()
or withKey()
.// Delete and return a Shopping List
ShoppingList deletedShoppingList = apiRoot
.shoppingLists()
.withId("{shoppingListID}")
.delete()
.withVersion(1l)
.withDataErasure(true) // Include to erase related personal data
.executeBlocking()
.getBody();
Retrieve the raw API response
.executeBlocking()
to return the resource as an instance of an object..sendBlocking()
. Note that with this approach you must create a byte array as the value to return:// Return a ShoppingListPagedQueryResponse as a byte array
byte[] shoppingLists = apiRoot.shoppingLists().get().sendBlocking().getBody();
// Convert to a String and output to the console
String shoppingListDetails = new String(
shoppingLists,
java.nio.charset.StandardCharsets.UTF_8
);
System.out.println(shoppingListDetails);
Use GraphQL
The response types have all available fields, but only the projected will contain a value.
final GraphQLRequest<ProductQueryResult> productQuery = GraphQL
.products(query -> query.localeProjection(Collections.singletonList("en")))
.projection(root ->
root.results().id().key().productType().key().getParent().createdAt()
);
final ApiHttpResponse<GraphQLResponse<ProductQueryResult>> response =
projectRoot.graphql().query(productQuery).executeBlocking();
final ProductQueryResult data = response.getBody().getData();
Use non-blocking calls
.execute()
. To return a byte array use .send()
:// Return all Shopping Lists using a CompletableFuture - execute()
apiRoot
.shoppingLists()
.get()
.execute()
.thenAccept(response -> {
ShoppingListPagedQueryResponse allShoppingLists = response.getBody();
// Assign the first Shopping List's ID to a string and output it to the console
String firstshoppingListID = allShoppingLists.getResults().get(0).getId();
System.out.println(firstshoppingListID);
})
.join();
// Return all Shopping Lists in a byte array using a CompletableFuture - send()
apiRoot
.shoppingLists()
.get()
.send()
.thenAccept(response -> {
byte[] shoppingLists = response.getBody();
// Convert to a String and output to the console
String shoppingListDetails = new String(
shoppingLists,
java.nio.charset.StandardCharsets.UTF_8
);
System.out.println(shoppingListDetails);
})
.join();