Learn how to set up and use the .NET SDK.
This step-by-step guide leads you through setting up and making API calls using the .NET SDK.
Requirements
To follow this guide you should have the following:
- A commercetools Composable Commerce Project
- An API Client
- .NET Standard 2.1 (or later)
Objectives of the get started guide
By the end of this guide you will have:
Placeholder values
Example code in this guide uses the following placeholder values. You should replace these placeholders with the following values.
Placeholder | Replace with | From |
---|---|---|
{projectKey} | project_key | your API Client |
{clientID} | client_id | your API Client |
{clientSecret} | secret | your API Client |
{scope} | scope | your API Client |
{region} | your Region | Hosts |
Install the .NET SDK
PackageReference
<ItemGroup>
<PackageReference Include="commercetools.Sdk.Api" Version="*" />
<PackageReference Include="commercetools.Sdk.ImportApi" Version="*" />
<PackageReference Include="commercetools.Sdk.HistoryApi" Version="*" />
</ItemGroup>
*
with the version number.commercetools.Sdk.Api
to use the Composable Commerce HTTP API. You need commercetools.Sdk.ImportApi
(Import API) and commercetools.Sdk.HistoryApi
(Change History API) only for more specific use cases.Set up the client
Add the following code to your Program:
// Include the following imports:
// using commercetools.Sdk.Api;
// using commercetools.Sdk.Api.Client;
// using Microsoft.Extensions.Configuration;
// using Microsoft.Extensions.DependencyInjection;
var services = new ServiceCollection();
var configuration = new ConfigurationBuilder()
.AddInMemoryCollection(new List<KeyValuePair<string, string>>()
{
new KeyValuePair<string, string>("MyClient:ApiBaseAddress", "https://api.{region}.commercetools.com/"),
new KeyValuePair<string, string>("MyClient:AuthorizationBaseAddress", "https://auth.{region}.commercetools.com/"),
new KeyValuePair<string, string>("MyClient:ClientId", "{clientID}"),
new KeyValuePair<string, string>("MyClient:ClientSecret", "{clientSecret}"),
new KeyValuePair<string, string>("MyClient:ProjectKey", "{projectKey}"),
new KeyValuePair<string, string>("MyClient:Scope", "{scope}")
})
.Build();
services.UseCommercetoolsApi(configuration, "MyClient");
services.AddLogging();
var serviceProvider = services.BuildServiceProvider();
var projectApiRoot = serviceProvider.GetService<ProjectApiRoot>();
projectApiRoot
to build requests to the Composable Commerce API. The following code makes an API call that gets your Project and assigns it to themyProject
variable. The Project's name is then output to the console using myProject.Name
.// Make a call to get the Project
var myProject = projectApiRoot
.Get()
.ExecuteAsync()
.Result;
// Output the Project name
Console.WriteLine(myProject.Name);
Use the .NET SDK
Imports
Without importing resource-specific types/namespaces you cannot use specific objects and methods.
For example, to create a Shopping List you must import:
using commercetools.Sdk.Api.Models.ShoppingLists;
Code examples within this guide display commented-out imports, where necessary.
Use builders
The .NET SDK follows a builder pattern when constructing drafts, update actions, and other objects/types that contain multiple fields.
// Include the following imports:
// using commercetools.Sdk.Api.Models.Categories;
// using commercetools.Sdk.Api.Models.Common;
// Create a LocalizedString
LocalizedString multiLanguageString = new LocalizedString(){
{"en", "English value"},
{"de", "German value"}
};
// Create US$100.00
Money money = new Money()
{
CurrencyCode = "USD",
CentAmount = 10000
};
// Create a Category
CategoryDraft categoryDraft = new CategoryDraft()
{
Name = new LocalizedString() { { "en", "english name" } },
Slug = new LocalizedString() { { "en", "english-slug" } },
Key = "category-key"
};
Structure your API call
Add an endpoint
projectApiRoot
. The following targets the Shopping Lists endpoint:var shoppingListInfo = projectApiRoot
.ShoppingLists()
// ...
If your IDE supports auto-complete, you can see the full list of endpoints.
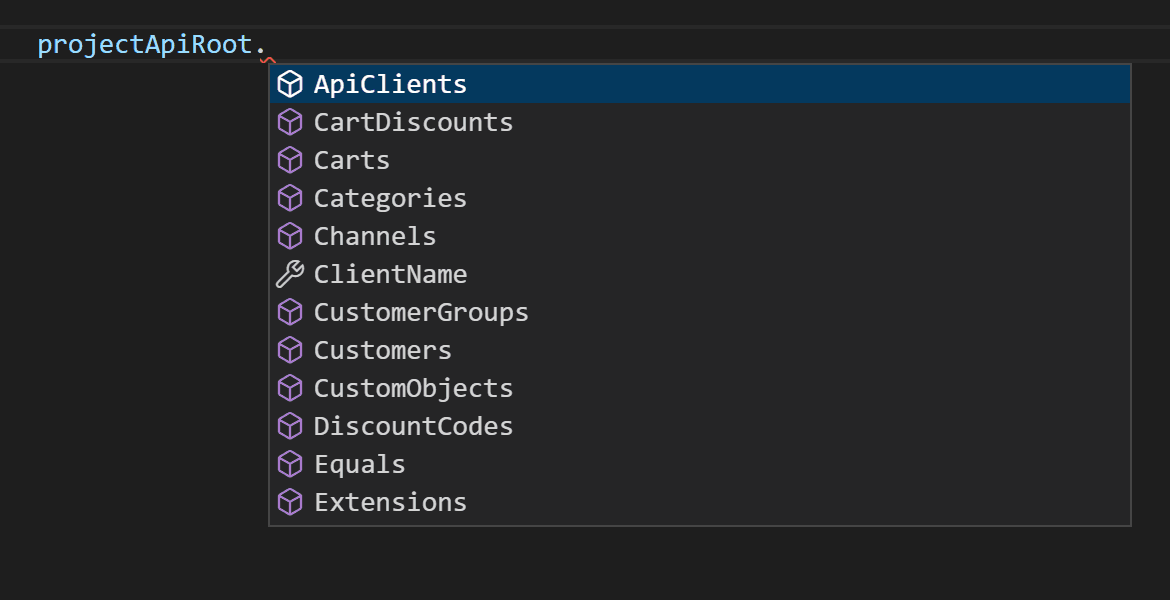
Retrieve data
Get a single resource
Get()
, ExecuteAsync()
, and Result
.// Get a specific Shopping List by ID
var shoppingListInfo = projectApiRoot
.ShoppingLists()
.WithId("a-shoppinglist-id")
.Get()
.ExecuteAsync()
.Result;
// Get a specific Shopping List by key
var shoppingListInfo = projectApiRoot
.ShoppingLists()
.WithKey("a-shoppinglist-key")
.Get()
.ExecuteAsync()
.Result;
shoppingListInfo
now contains the data of the specified Shopping List. You can access information from the fields within that object: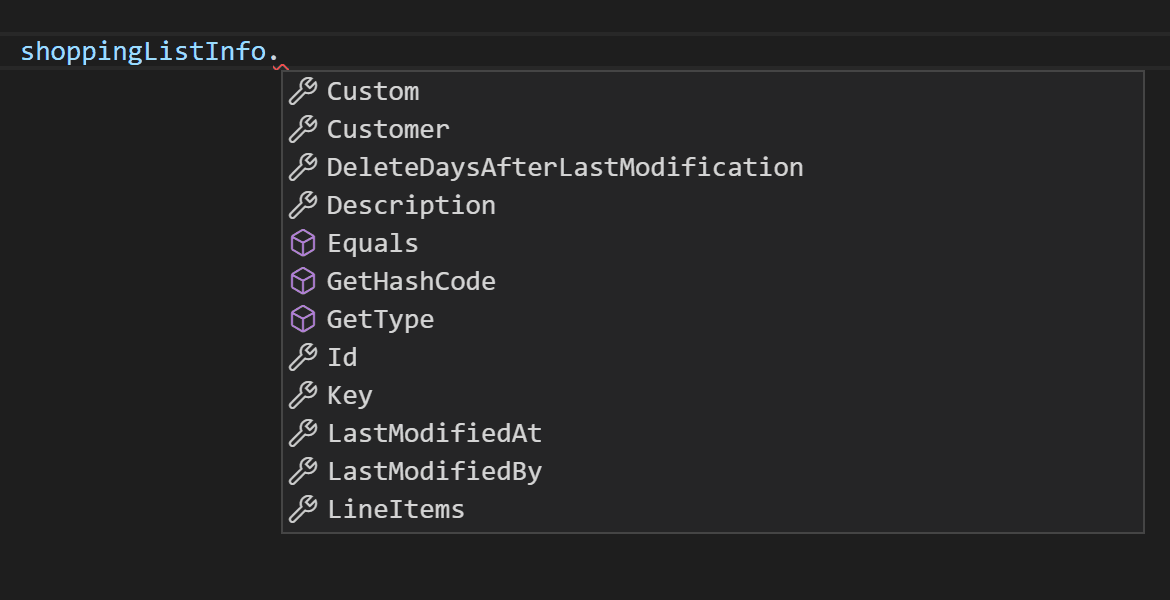
Get multiple resources
PagedQueryResponse
, which is identical to PagedQueryResults in the HTTP API.// Return an IShoppingListPagedQueryResponse
var shoppingListsQuery = projectApiRoot
.ShoppingLists()
.Get()
.ExecuteAsync()
.Result;
WithWhere()
, WithSort()
, WithExpand()
, WithLimit()
, or WithOffset()
after Get()
.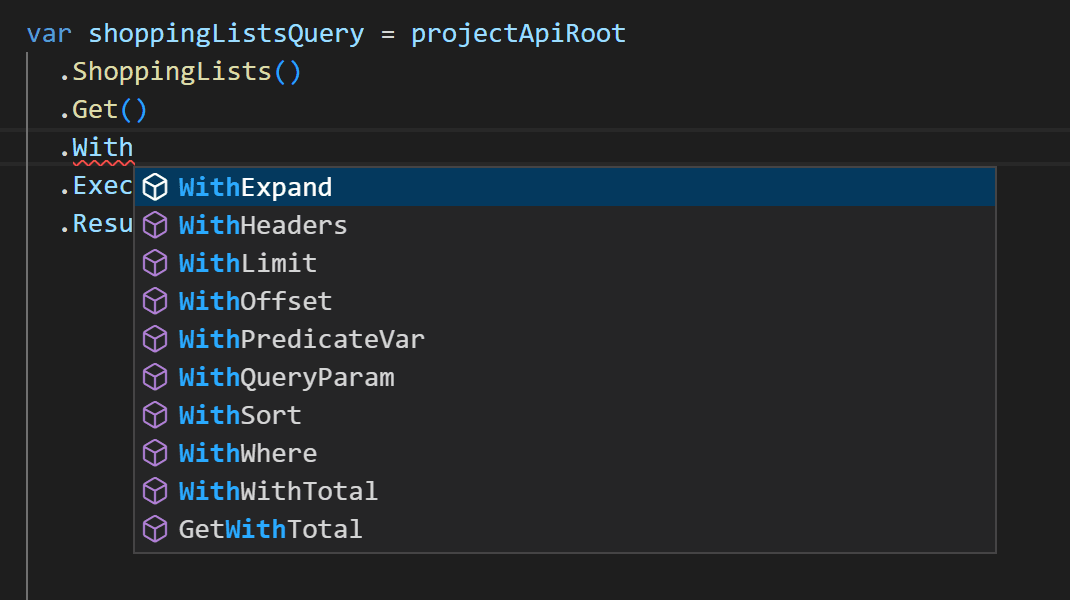
Use the Query Predicate builder
withQuery
method.// Return all Customers that have not verified their email address
var response = projectApiRoot
.Customers()
.Get()
.WithQuery(c => c.IsEmailVerified().Is(false))
.ExecuteAsync()
.Result;
View results
PagedQueryResponse
using Result
:// Return an IShoppingListPagedQueryResponse
var shoppingListsQuery = projectApiRoot
.ShoppingLists()
.Get()
.ExecuteAsync()
.Result;
// Put the returned Shopping Lists in a list
var listOfShoppingLists = shoppingListsQuery.Results;
// Output the first Shopping List's English name
Console.WriteLine(listOfShoppingLists[0].Name["en"]);
Write a resource
Create a new resource
// Build a ShoppingListDraft with the required fields (name)
var newShoppingListDetails = new ShoppingListDraft()
{
Name = new LocalizedString() { { "en", "English name of Shopping List" } }
};
newShoppingListDetails
within Post()
and follow it with ExecuteAsync()
.// Post the ShoppingListDraft and get the new Shopping List
var newShoppingList = projectApiRoot
.ShoppingLists()
.Post(newShoppingListDetails)
.ExecuteAsync()
.Result;
Update an existing resource
ShoppingListUpdate
) contains a collection of update actions and the last seen version of the resource.// Build a ShoppingListUpdate with the required fields (version and list of IShoppingListUpdateActions)
var shoppingListUpdate = new ShoppingListUpdate()
{
Version = 1,
Actions = new List<IShoppingListUpdateAction>
{
{
new ShoppingListSetKeyAction(){Key="a-unique-shoppinglist-key"}
}
}
};
WithId()
or WithKey()
).// Post the ShoppingListUpdate and return the updated Shopping List
var updatedShoppingList = projectApiRoot
.ShoppingLists()
.WithId("{shoppingListID}")
.Post(shoppingListUpdate)
.ExecuteAsync()
.Result;
Delete a resource
.Delete()
method with the last seen version of the resource. You must identify the resource to delete using WithId()
or WithKey()
.// Delete and return a Shopping List
var deletedShoppingList = projectApiRoot
.ShoppingLists()
.WithId("{shoppingListID}")
.Delete()
.WithVersion(1)
.WithDataErasure(true) // Include to erase related personal data
.ExecuteAsync()
.Result;
Use GraphQL
The response types will have all available fields defined by the selector.
var variables = new { productFilter = $@"id = ""{productId}""" };
var response = await client.Query(variables,
static (i, o) => o.Products(where: i.productFilter,
selector: r => new { results = r.Results(product => new { product.Id })}
)
);
Assert.NotNull(response.Data?.results[0].Id);