Learn how to set up and use the TypeScript SDK.
This step-by-step guide leads you through setting up and making API calls using the TypeScript SDK.
Requirements
To follow this guide you should have the following:
- A commercetools Composable Commerce Project
- An API Client
- Node v18.17.x (or later)
- Either
npm
v9.6.x (or later) oryarn
v3.2.x (or later)
Objectives of the get started guide
By the end of this guide you will have:
Placeholder values
Example code in this guide uses the following placeholder values. You should replace these placeholders with the following values.
Placeholder | Replace with | From |
---|---|---|
{projectKey} | project_key | your API Client |
{clientID} | client_id | your API Client |
{clientSecret} | secret | your API Client |
{scope} | scope | your API Client |
{region} | your Region | Hosts |
Install the TypeScript SDK
Use the following commands to install SDK components from npm or Yarn.
SDK | npm | Yarn |
---|---|---|
SDK Client | npm install @commercetools/ts-client | yarn add @commercetools/ts-client |
HTTP API | npm install @commercetools/platform-sdk | yarn add @commercetools/platform-sdk |
Import API | npm install @commercetools/importapi-sdk | yarn add @commercetools/importapi-sdk |
Audit Log API | npm install @commercetools/history-sdk | yarn add @commercetools/history-sdk |
Create a ClientBuilder file
BuildClient.ts
and insert the following code.This code creates a Client for making API calls.
import fetch from 'node-fetch';
import {
ClientBuilder,
// Import middlewares
type AuthMiddlewareOptions, // Required for auth
type HttpMiddlewareOptions, // Required for sending HTTP requests
} from '@commercetools/ts-client';
const projectKey = '{projectKey}';
const scopes = ['{scope}'];
// Configure authMiddlewareOptions
const authMiddlewareOptions: AuthMiddlewareOptions = {
host: 'https://auth.{region}.commercetools.com',
projectKey: projectKey,
credentials: {
clientId: '{clientID}',
clientSecret: '{clientSecret}',
},
scopes,
httpClient: fetch,
};
// Configure httpMiddlewareOptions
const httpMiddlewareOptions: HttpMiddlewareOptions = {
host: 'https://api.{region}.commercetools.com',
httpClient: fetch,
};
// Export the ClientBuilder
export const ctpClient = new ClientBuilder()
.withProjectKey(projectKey) // .withProjectKey() is not required if the projectKey is included in authMiddlewareOptions
.withClientCredentialsFlow(authMiddlewareOptions)
.withHttpMiddleware(httpMiddlewareOptions)
.withLoggerMiddleware() // Include middleware for logging
.build();
Add middleware
authMiddlewareOptions
and httpMiddlewareOptions
to handle auth and HTTP requests respectively.ctpClient
with method chaining.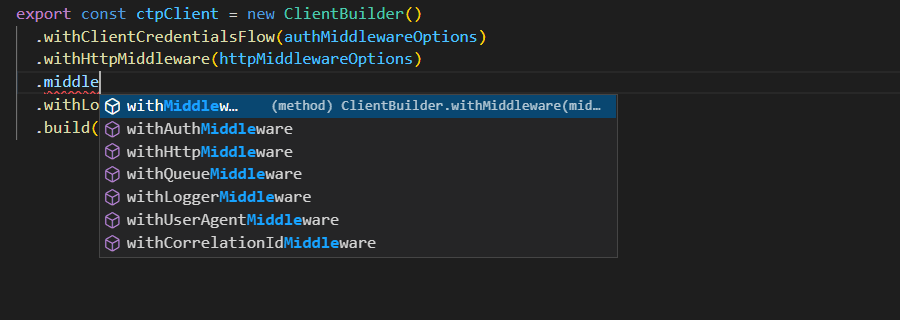
Create the Client
Create a new file and include the following code:
import { ctpClient } from './BuildClient';
import {
ApiRoot,
createApiBuilderFromCtpClient,
} from '@commercetools/platform-sdk';
// Create apiRoot from the imported ClientBuilder and include your Project key
const apiRoot = createApiBuilderFromCtpClient(ctpClient)
.withProjectKey({ projectKey: '{projectKey}' });
// Example call to return Project information
// This code has the same effect as sending a GET request to the commercetools Composable Commerce API without any endpoints.
const getProject = () => {
return apiRoot
.get()
.execute();
};
// Retrieve Project information and output the result to the log
getProject()
.then(console.log)
.catch(console.error);
createApiBuilderFromCtpClient
function accepts an optional second argument called baseUri
, which is of type string.
You can use this argument to override the host parameters in httpMiddlewareOptions
.createApiBuilderFromCtpClient(client: Client, baseUri?: string): ApiRoot;
apiRoot
to build requests to the Composable Commerce API.getProject()
as an example. Running this code returns the Project.Structure your API call
Add an endpoint
apiRoot
. The following targets the Shopping List endpoint:apiRoot
.shoppingLists();
If your IDE supports auto-complete, you can see the full list of endpoints.
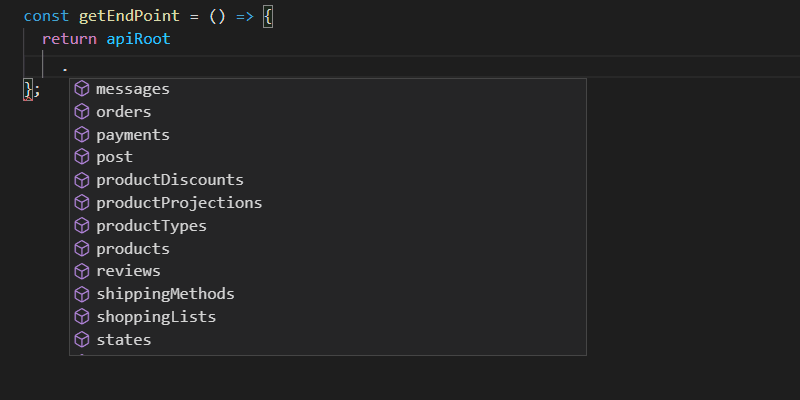
Query a specific entity using withID() or withKey()
id
or key
using .withId()
or .withKey()
respectively.apiRoot
.shoppingLists()
.withId({ ID: 'a-shoppinglist-id' });
apiRoot
.shoppingLists()
.withKey({ key: 'a-shoppinglist-key' });
Add the method
After selecting the endpoint, select a method to use.
get()
to query entities, post()
to create and update entities, and .delete()
to delete entities.apiRoot
.shoppingLists()
.withId({ ID: 'a-shoppinglist-id' })
.get();
Add parameters and payloads
get()
. When creating and updating entities, include parameters and payloads within post()
.When getting the Shopping List endpoint, you can include optional parameters to modify what Shopping Lists are returned:
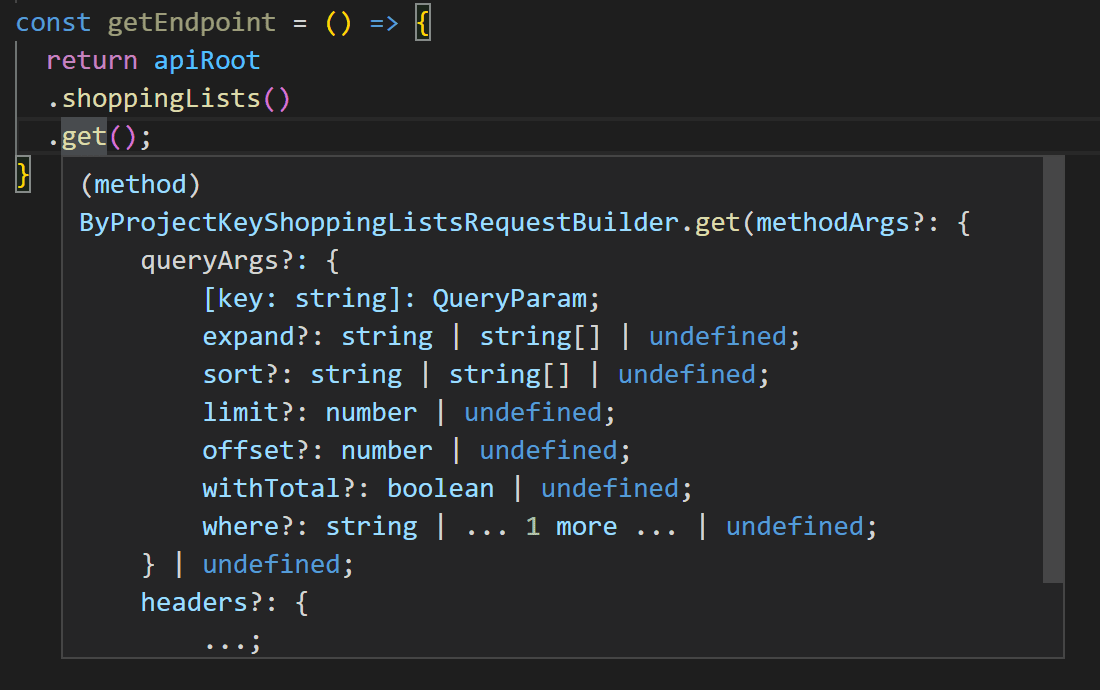
body
payload. This payload (ShoppingListDraft) creates a new Shopping List: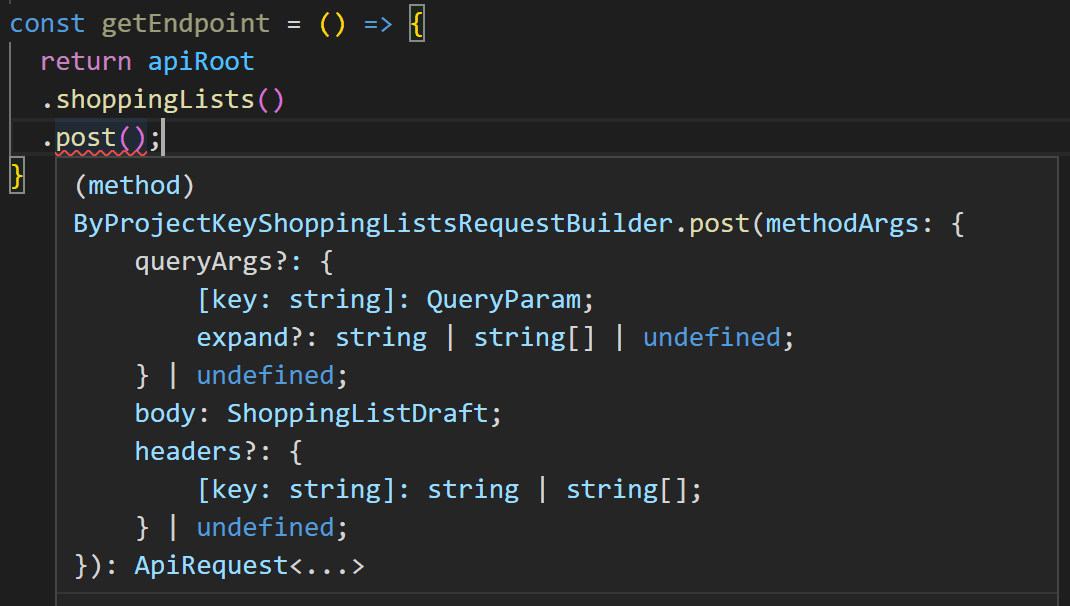
body
payload. This payload (ShoppingListUpdate
) updates the specified Shopping List: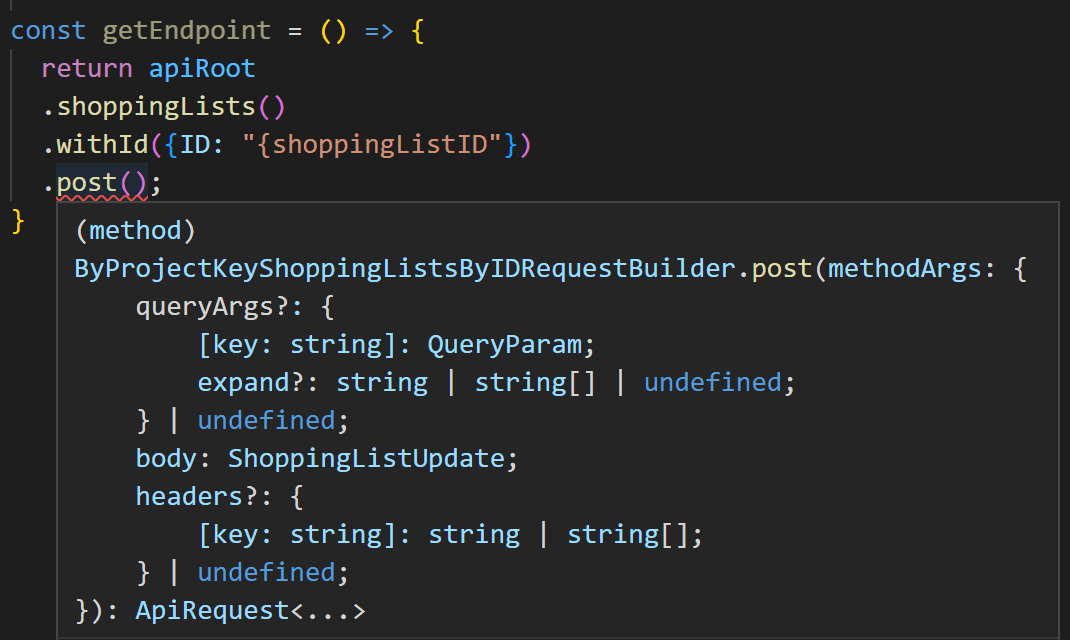
.execute() .then() .catch()
.execute()
sends the API request..then()
adds functionality that should follow the API request. In the following example code, then()
sends the response to the console..catch()
assists with debugging and error handling.apiRoot
.shoppingLists()
.withId({ ID: 'a-shoppinglist-id' })
.get()
.execute()
.then(({ body }) => {
console.log(JSON.stringify(body));
})
.catch(console.error);
Use the TypeScript SDK in the browser
You can use the TypeScript SDK as an embedded Universal Module Definition (UMD) module or an imported package in a frontend framework/library such as React.
As an embedded UMD module
Create an HTML file and insert the following code:
<!DOCTYPE html>
<html lang="en">
<head>
<title>TypeScript SDK Examples</title>
<script src="https://unpkg.com/browse/@commercetools/ts-client@latest/dist/commercetools-ts-client.umd.js"></script>
<script src="https://unpkg.com/@commercetools/platform-sdk@latest/dist/commercetools-platform-sdk.umd.js"></script>
</head>
<body>
<!-- Click this button to return the Project details -->
<p>
<button onclick="getProjectDetails()">Get Project Information</button>
</p>
<!-- This text is overwritten when getProjectDetails() finishes -->
<p id="details">
Click the above button to display the Project information.
</p>
</body>
<script>
// Enter your API client configuration
var oauthUri = 'https://auth.{region}.commercetools.com';
var baseUri = 'https://api.{region}.commercetools.com';
var credentials = {
clientId: '{clientID}',
clientSecret: '{clientSecret}',
};
var projectKey = '{projectKey}';
// Builds the client
var { ClientBuilder } = window['@commercetools/ts-client'];
var client = new ClientBuilder()
.defaultClient(baseUri, credentials, oauthUri, projectKey)
.build();
var { createApiBuilderFromCtpClient } =
window['@commercetools/platform-sdk'];
var apiRoot = createApiBuilderFromCtpClient(client).withProjectKey({
projectKey,
});
// Returns the Project details
function getProjectDetails() {
apiRoot
.get()
.execute()
.then(function ({ body }) {
window.document.getElementById('details').innerHTML =
JSON.stringify(body);
});
}
</script>
</html>
When loaded in your web browser this page displays a button that, when clicked, returns your Project information.
getProjectDetails()
function is similar to code examples within this get started guide. Consult the previous code examples to add further functionality to the HTML document.As an imported package
App.tsx
file.import React, { useState, useEffect } from 'react';
import { ClientBuilder, type Client } from '@commercetools/ts-client';
import {
createApiBuilderFromCtpClient,
ApiRoot,
} from '@commercetools/platform-sdk';
const BASE_URI = 'https://api.{region}.commercetools.com';
const OAUTH_URI = 'https://auth.{region}.commercetools.com';
const PROJECT_KEY = '{projectKey}';
const CREDENTIALS = {
clientId: '{clientID}',
clientSecret: '{clientSecret}',
};
export function App() {
const [projectDetails, setProjectDetails] = useState({});
// Create client
const getClient = (): Client => {
return new ClientBuilder()
.defaultClient(BASE_URI, CREDENTIALS, OAUTH_URI, PROJECT_KEY)
.build();
};
// Get apiRoot
const getApiRoot = (client: Client): ApiRoot => {
return createApiBuilderFromCtpClient(client);
};
useEffect(function () {
const client = getClient();
const apiRoot = getApiRoot(client);
apiRoot
.withProjectKey({ projectKey: PROJECT_KEY })
.get()
.execute()
.then(({ body }) => {
setProjectDetails(body);
})
.catch(console.error);
}, []);
return (
<div>
<h2>Project details for {PROJECT_KEY}:</h2>
<pre>{JSON.stringify(projectDetails, null, 2)}</pre>
</div>
);
}