To implement a guest checkout, you can assign Carts and Orders to an anonymous session, and later assign those to a Customer account on sign in or sign up. This tutorial teaches you how, both with special OAuth access tokens and the /me
endpoints, and with regular tokens. To follow this tutorial, you should have a Project set up, as described in Getting Started. If you want to use the special OAuth access tokens, you should know how to work with Password Flow tokens as described in this tutorial.
Identifier for Anonymous Sessions
Carts and Orders have a field anonymousId
that define to which Anonymous Session they belong. This identifier can be freely chosen, and you could re-use an identifier given to you by an analytics library or a Device ID on a mobile device. However, if you do not use the OAuth access tokens to manage the session for you, you need to make sure that no other session with that identifier already exists.
Use Anonymous Sessions with special OAuth tokens
Using OAuth tokens has the advantage that carts created with the My Carts endpoint are automatically assigned to the session, and that the My Customers Profile endpoint assigns the carts and orders automatically to the customer account during sign up and sign in.
Create an OAuth client
To create a token for an Anonymous Session, the OAuth client needs the create_anonymous_token
permission. For this tutorial, we need to create an OAuth client that also has the permissions view_products
, manage_my_orders
and manage_my_profile
. In the Merchant Center, go to the "Developer Settings" section and create a new API Client with the template "Mobile client" that contains the required permissions.
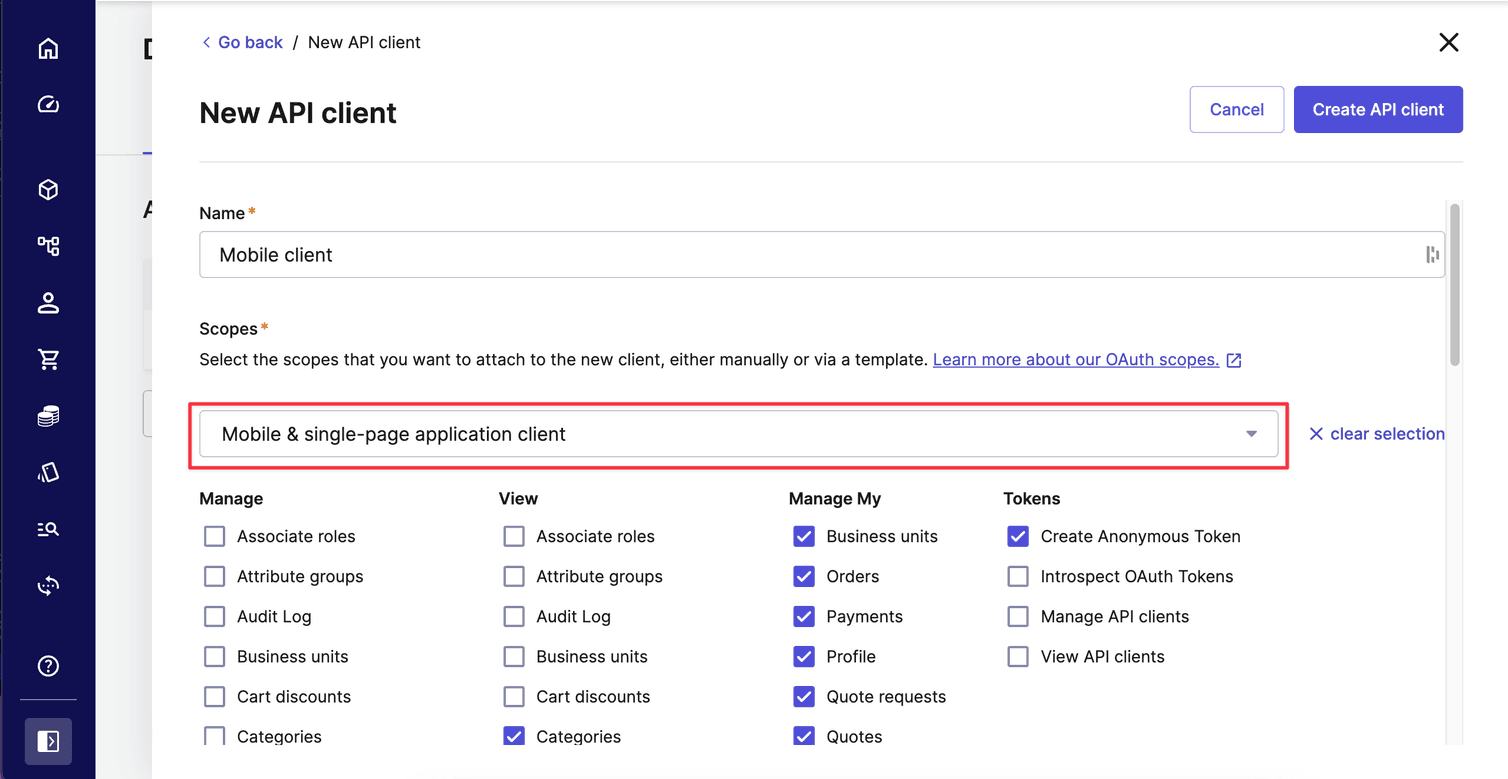
Copy or download the client credentials, they cannot be revealed after creation for security reasons.
Create a token with a new Anonymous Session
We use the OAuth client we just created to get a token. Note that we need the create_anonymous_token
permission only to create the token, but it's not necessary anymore once the token has been created. In this example, we let the API generate an identifier for us.
$curl https://auth.{region}.commercetools.com/oauth/{projectKey}/anonymous/token --basic --user "{client_id}:{client_secret}" -X POST -d "grant_type=client_credentials&scope=view_products:{projectKey} manage_my_orders:{projectKey} manage_my_profile:{projectKey}"
In this example, we supply the identifier phone-123
. If this identifier is already in use, the request will fail.
$curl https://auth.{region}.commercetools.com/oauth/{projectKey}/anonymous/token --basic --user "{client_id}:{client_secret}" -X POST -d "grant_type=client_credentials&scope=view_products:{projectKey} manage_my_orders:{projectKey} manage_my_profile:{projectKey}&anonymous_id=phone-123"
The response contains the token in the field access_token
:
{
"access_token": "vkFuQ6oTwj8_Ye4eiRSsqMeqLYNeQRJi",
"token_type": "Bearer",
"expires_in": 172800,
"refresh_token": "{projectKey}:OWStLG0eaeVs7Yx3-mHcn8iAZohBohCiJSDdK1UCJ9U",
"scope": "view_products:{projectKey} manage_my_orders:{projectKey} manage_my_profile:{projectKey}"
}
This token can now be used to access products, for example:
$curl -sH "Authorization: Bearer vkFuQ6oTwj8_Ye4eiRSsqMeqLYNeQRJi" https://api.{region}.commercetools.com/{projectKey}/product-projections?staged=true&limit=10
Best practice: Create Anonymous Sessions only once necessary
Refer to the best practice on when to create a new anonymous session.
Use the token to create a cart and order
We can create a new cart that belongs to the Anonymous Session:
POST /{projectKey}/me/carts
with:
{
"currency": "USD",
"country": "US"
}
The new cart will have the anonymousId
automatically set, for example to phone-123
.
We can now use this cart much like we're using any other cart, for example we can add a line item:
POST /{projectKey}/me/carts/<cart-id>
with:
{
"version": <cart-version>,
"actions": [{
"action": "addLineItem",
"productId": "<product-id>",
"variantId": 1,
"quantity": 1
}]
}
We can also order the cart. The new order will also automatically belong to the Anonymous Session, for example the anonymousId
will be phone-123
.
Keep the refresh token
With the refresh token, you can get new access tokens for the Anonymous Session. It can live for a long time, and contain as many carts and orders as you need. However, if the refresh token is lost, there's no other way to claim this Anonymous Session.
Depending on your platform, you can save the refresh token for later usage. For example, a browser app may save it in local storage, or an iOS app in the keychain.
End an Anonymous Session by assigning it to a Customer
If a customer signs up or signs in, the carts and orders belonging to the Anonymous Session are automatically assigned to the customer account. The field anonymousId
will still be set. After the session has ended, the access token and refresh token are immediately invalid.
In this example, a customer signs up. After we have collected important information like name, email, and password from the customer, we can send their data to the API:
POST /{projectKey}/me/signup
with:
{
"firstName": "Alice",
"lastName": "Doe",
"email": "alice@example.com",
"password": "secret"
}
Now we need to get new access and refresh tokens with the password flow:
$curl https://auth.{region}.commercetools.com/oauth/{projectKey}/customers/token --basic --user "{client_id}:{client_secret}" -X POST -d "grant_type=password&username=alice@example.com&password=secret&scope=view_products:{projectKey} manage_my_orders:{projectKey} manage_my_profile:{projectKey}"
A GET /{projectKey}/me/carts
with the new access token returns the same carts, but we'll notice that the customerId
is the one of the newly created account. The anonymousId
is still set to phone-123
. If we create another cart, it will have the customerId
set, but not the anonymousId
.
Use Anonymous Sessions with regular OAuth tokens
Using regular OAuth tokens, you need to create the identifier yourself (and make sure it has not been used yet). You can assign it to a cart during creation:
POST /{projectKey}/carts
with:
{
"currency": "USD",
"country": "US",
"anonymousId": "foobar"
}
If this cart is ordered, the anonymousId
will also be set on the new order.
To obtain all carts belonging to the Anonymous Session, we can use the Predicate anonymousId = "foobar"
:
GET /{projectKey}/carts?where=anonymousId%20%3D%20%22foobar%22
When the customer signs in or up, you need to provide the anonymousId
in the request. This is an example for sign up:
POST /{projectKey}/customers
with:
{
"firstName": "Alice",
"lastName": "Doe",
"email": "alice@example.com",
"password": "secret",
"anonymousId": "foobar"
}
All carts and orders with the anonymousId
foobar
are assigned to the newly created customer account.
Conclusion
You've seen how to use Anonymous Sessions both with special tokens and the /me
endpoints, and with regular tokens. Now you can implement guest checkout easily in your application!