Learn how to initialize and customize Checkout.
After completing this page, you should be able to:
- Identify customization possibilities by referring to the Checkout documentation.
The Checkout SDK simplifies the integration process. Here's a step-by-step guide to initialize Checkout using JSFiddle.
Steps
-
Create a Cart with
Lineitems
in it. You can use Postman or the Merchant Center to create a Cart with Items in it. -
Create a Checkout Session. Utilize the Sessions endpoint to create a session for the Cart, obtaining a Session ID.
-
Initialize the SDK by updating your HTML code with your Project key, Region, Session ID, and locale. In this demo we are using the checkoutFlow method. If you want to reuse your own checkout and our payment integrations, use the paymentFlow method.
The following is sample HTML code for
checkoutFlow
:
<!DOCTYPE html>
<html>
<head>
<title>Checkout Example</title>
<script>
(function (w, d, s) {
if (w.ctc) {
return;
}
var js,
fjs = d.getElementsByTagName(s)[0];
var q = [];
w.ctc =
w.ctc ||
function () {
q.push(arguments);
};
w.ctc.q = q;
js = d.createElement(s);
js.type = 'text/javascript';
js.async = true;
js.src =
'https://unpkg.com/@commercetools/checkout-browser-sdk@latest/browser/sdk.js';
fjs.parentNode.insertBefore(js, fjs);
})(window, document, 'script');
ctc('checkoutFlow', {
projectKey: '{product-key}',
region: '{region}',
sessionId: '{session-id}',
locale: 'en', // Optional: specify the desired locale
logInfo: true, // Recommended for debugging during development.
logWarn: true, // Recommended for debugging during development.
logError: true, // Recommended for debugging during development.
});
</script>
</head>
<body>
<h1>Checkout Example</h1>
<div data-ctc></div>
</body>
</html>
- Execute the code. The code must be loaded from a public domain with HTTPS enabled; this is needed to support cross-origin resource sharing (CORS). Tools that can help you enable this include Ngrok, Pinggy, or a browser code editor like JSFiddle.
- Ensure that you have the origin URL (from step 4) added to the Checkout Application. If you are using JS fiddle, then you will need to add https://jsfiddle.net and https://fiddle.jshell.net. This setting allows us to resolve CORS.
Remember, in the preceding sample code, you need to update the highlighted lines (27 to 31). Once you run the code, you should see a default themed checkout like the following:
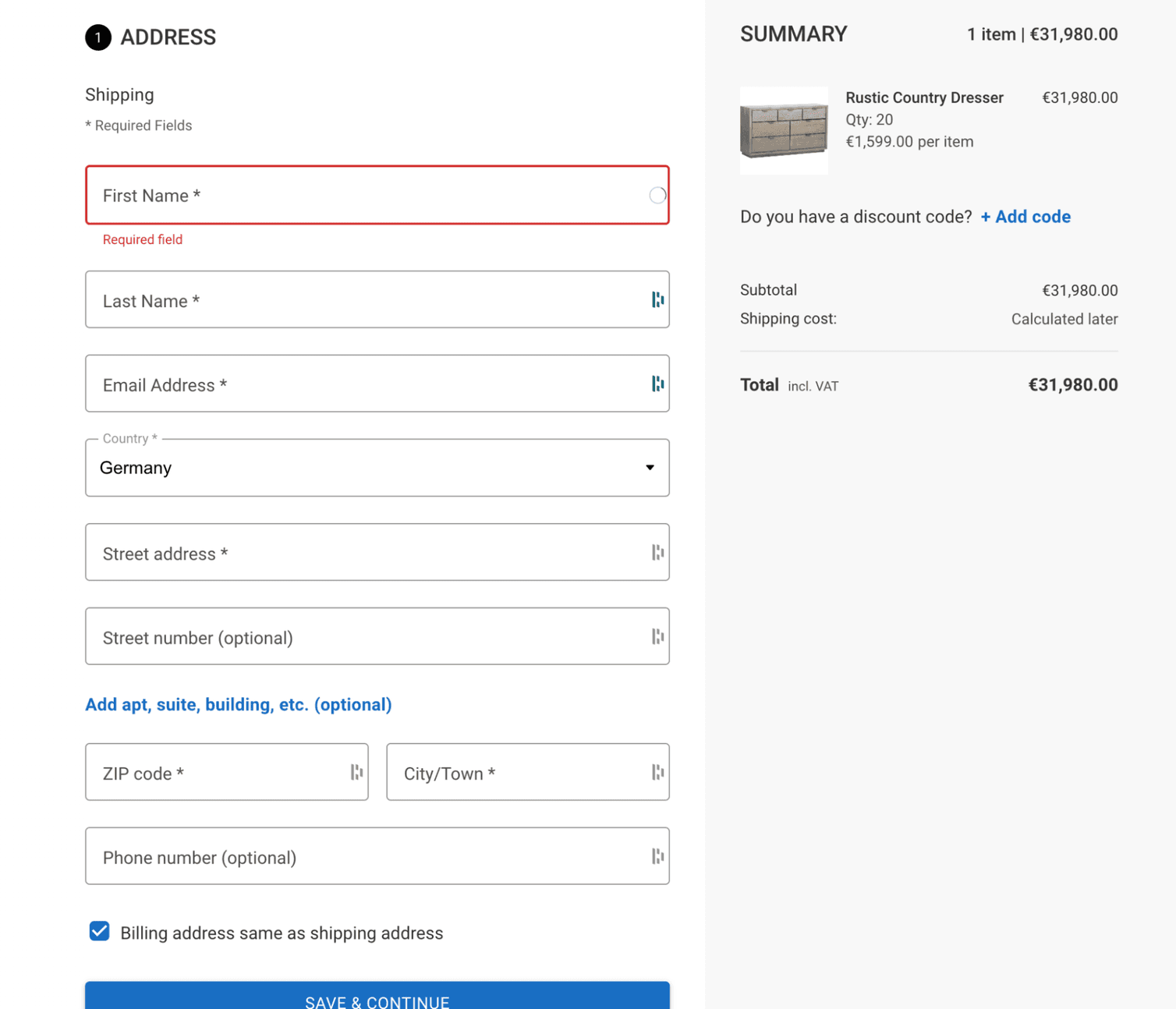
Payment Only mode
Let's now look at the small difference we need to make if we use the Payment Only mode. Here is some sample HTML code, where the only change is that checkoutFlow
is swapped with paymentFlow
.
<!DOCTYPE html>
<html>
<head>
<title>Checkout Example</title>
<script>
(function (w, d, s) {
if (w.ctc) {
return;
}
var js,
fjs = d.getElementsByTagName(s)[0];
var q = [];
w.ctc =
w.ctc ||
function () {
q.push(arguments);
};
w.ctc.q = q;
js = d.createElement(s);
js.type = 'text/javascript';
js.async = true;
js.src =
'https://unpkg.com/@commercetools/checkout-browser-sdk@latest/browser/sdk.js';
fjs.parentNode.insertBefore(js, fjs);
})(window, document, 'script');
ctc('paymentFlow', {
projectKey: '{product-key}',
region: '{region}',
sessionId: '{session-id}',
locale: 'en', // Optional: specify the desired locale
logInfo: true, // Recommended for debugging during development.
logWarn: true, // Recommended for debugging during development.
logError: true, // Recommended for debugging during development.
});
</script>
</head>
<body>
<h1>Checkout Example</h1>
<div data-ctc></div>
</body>
</html>
Payment Only mode is initialized, we should expect to see only the payment methods rendered, as in the following screenshot:
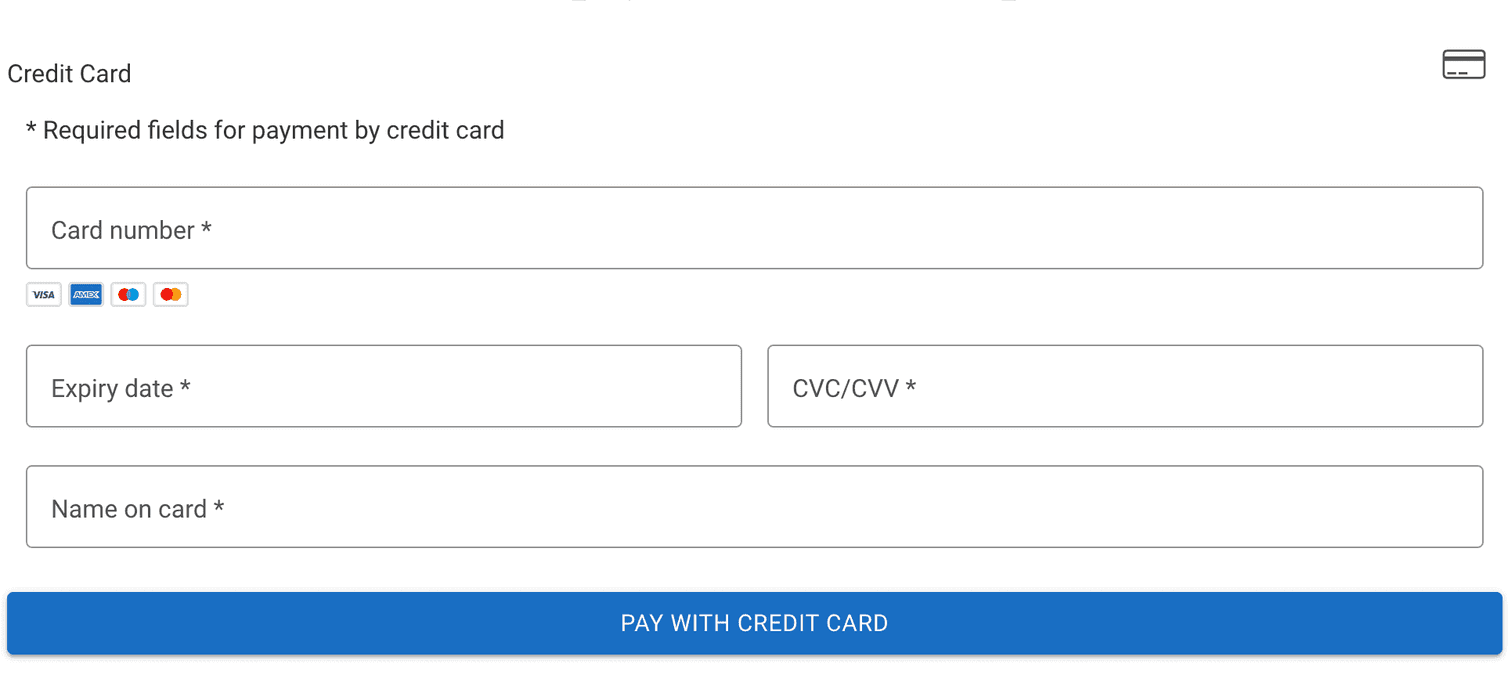
Sample payment Connector test card
If you're using the sample payment Connector, you can test payments with a sample credit card. We provide sample credit card details here.
Checkout customizations
Now that you have Checkout running, you can customize the checkout experience to align with your brand:
- Styling: apply custom CSS to match your brand identity.
- Texts and labels: modify texts and labels for a localized experience.
- Address forms: customize address forms for specific regions.
- Address validation: implement custom address validation rules.