Discover common components to help build the foundation of your user interface.
Developing a customization requires you to implement a user interface (UI). To facilitate the development of UI features, we provide ready-to-use components based on the commercetools design system, UI Kit. These components provide users of your customizations with a user interface similar to the built-in applications of the Merchant Center, ensuring a consistent and familiar user experience.
Spacings components
To position elements within your customization, use the <Spacings>
components. These components use dedicated spacing values to ensure a consistent and familiar user experience across all applications in the Merchant Center.
The two primary variants of the <Spacings>
components: Stack
and Inline
.
You can think about these variants in the following way:
Stack
: stacks elements vertically.Inline
: arranges elements horizontally.
The <Spacings>
components are implemented using CSS Flexbox. This makes it simple to align children of these elements together and control the spacing between them.
Let's say we are implementing a List Page, which is a page to show a list of a specific resource.
A simple layout might look something like this:
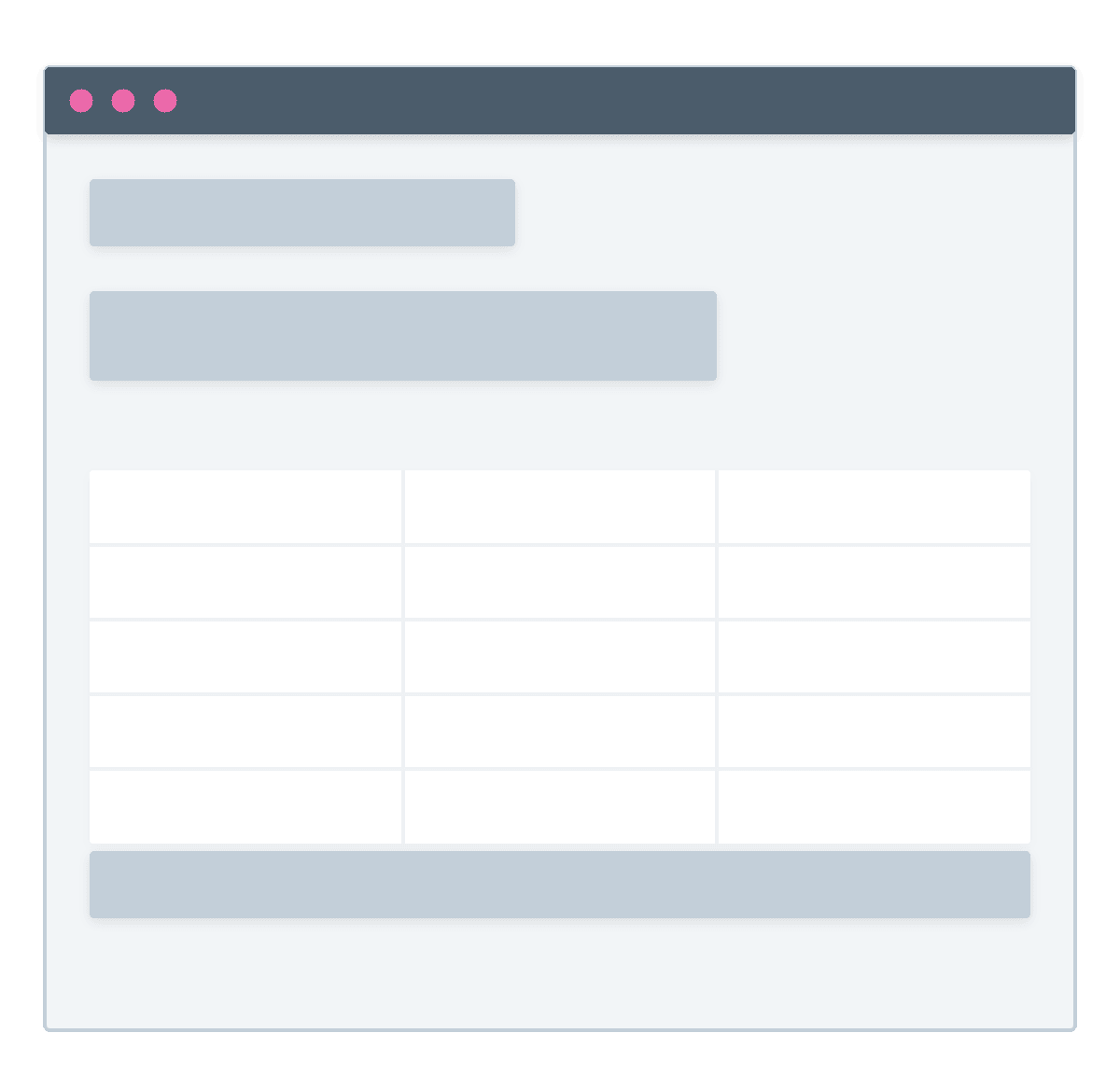
Because most of the elements are vertically stacked, we can use the Stack
component.
import Spacings from '@commercetools-uikit/spacings';
const Channels = () => {
// ...
return (
<Spacings.Stack>
<div>Title</div>
<div>Description</div>
<table>{/* Table data */}</table>
<div>{/* Pagination */}</div>
</Spacings.Stack>
);
};
By default, the Stack
component has a default scale
of s
. The scale
determines the space between each child.
In our Channels
component example, we want to have different amounts of space between individual components. To do this, we can nest multiple Stack
components with different scale
values.
import Spacings from '@commercetools-uikit/spacings';
const Channels = () => {
// ...
return (
<Spacings.Stack scale="l">
<Spacings.Stack scale="s">
<div>Title</div>
<div>Description</div>
</Spacings.Stack>
<Spacings.Stack scale="xs">
<table>{/* Table data */}</table>
<div>{/* Pagination */}</div>
</Spacings.Stack>
</Spacings.Stack>
);
};
The changes we made can be translated as:
- The outer elements have a
scale
ofl
, which provides a relatively large amount of space in between. - The title and description have a
scale
ofs
, which makes them relatively close to each other. - The table and pagination have a
scale
ofxs
, which makes them very close to each other.
Using these principles, you can use as many Stack
components as is necessary to define your vertical layout.
The same principles apply to the Inline
components.
Typography
To render text within your customization, use the <Text>
component. This component uses dedicated typographic styles to ensure consistent typography across the Merchant Center.
The <Text>
component exposes the following variants:
Headline
andSubheadline
: for headline elements from H1 to H5.Body
: for normal content.Detail
: for smaller or secondary content.Caption
: for small descriptive labels that provide additional information in a given context.
Let's add these styles to our example Channels
component:
import Spacings from '@commercetools-uikit/spacings';
import Text from '@commercetools-uikit/text';
const Channels = () => {
// ...
return (
<Spacings.Stack scale="l">
<Spacings.Stack scale="s">
<Text.Headline as="h2">Title</Text.Headline>
<Text.Body>Description</Text.Body>
</Spacings.Stack>
<Spacings.Stack scale="xs">
<table>{/* Table data */}</table>
<div>{/* Pagination */}</div>
</Spacings.Stack>
</Spacings.Stack>
);
};
Render data in tables
To display data using tables, use the <DataTable>
components.
To get started, you need to define the columns of the table.
const columns = [
{ key: 'name', label: 'Channel name' },
{ key: 'key', label: 'Channel key', isSortable: true },
{ key: 'roles', label: 'Roles' },
];
In this example, we define three columns with the key
as the identifier, a label
, and we make the key
column sortable.
Next, we render the table.
import Spacings from '@commercetools-uikit/spacings';
import Text from '@commercetools-uikit/text';
import DataTable from '@commercetools-uikit/data-table';
import { NO_VALUE_FALLBACK } from '@commercetools-frontend/constants';
const columns = [
{ key: 'name', label: 'Channel name' },
{ key: 'key', label: 'Channel key', isSortable: true },
{ key: 'roles', label: 'Roles' },
];
const itemRenderer = (item, column) => {
switch (column.key) {
case 'roles':
return item.roles.join(', ');
case 'name':
const localizedName = item.nameAllLocales.find(
(field) => field.locale === 'en'
);
return localizedName ?? NO_VALUE_FALLBACK;
default:
return item[column.key];
}
};
const Channels = () => {
// ...
return (
<Spacings.Stack scale="l">
<Spacings.Stack scale="s">
<Text.Headline as="h2">Title</Text.Headline>
<Text.Body>Description</Text.Body>
</Spacings.Stack>
<Spacings.Stack scale="xs">
<DataTable
columns={columns}
rows={channels.results}
itemRenderer={itemRenderer}
/>
<div>{/* Pagination */}</div>
</Spacings.Stack>
</Spacings.Stack>
);
};
The itemRenderer
is the main function used to render the table cell values. It can be a simple string or some React components.
The rows
field is the list of the actual data that needs to be rendered in the table.