modular_store
have a default UI theme that is set in the InStore Center. You can override the styling of this theme for the following product modules:- InStore_Payment
- InStore_Refund
- InStore_DeviceManagement
- InStore_CashManagement
You must add an API call to override styling defaults before these modules are called. You can override the following aspects of the front-end presentation of InStore's core modules:
Override the theme
-
Import the
InStore_State
module, formerly known as/store
:import { useStore } from "InStore_State/store";
-
Get the
configuration
from theuseStore
in your main component. For example, theInStore_Shell
module usesInStore_Shell/src/views/layout.tsx
.`const Layout = () => { const { configuration } = useStore();
-
Call the
setOverrideCoreTheme
parameter at any point before any of the InStore product modules are loaded. For example, thesetOverrideCoreTheme
is called on the mount of the main layout of theInStore_Shell
parameter.useEffect(() => { configuration.setOverrideCoreTheme(true) }, [])
-
If the
overrideCoreTheme
parameter is set astrue
, then the theme set onthemeOptions
in theInStore_Shell/src/providers/theme.tsx
file will be applied to the InStore product modules. For example:import React, { PropsWithChildren } from 'react' import { ThemeProvider as TP } from 'InStore_State/theme' import customTheme from '../theme/customTheme' const ThemeProvider = ({ children }: PropsWithChildren) => { return ( <TP rootElementId="app" themeOptions={customTheme}> {children} </TP> ) }
HTML structure for Material UI in product modules
HTML structure for payment types
<Grid className="instore-payment-entry-container">
<Grid item xs=12 md=6 className="instore-payment-amount-section">
<Grid item xs=12>
<Typography className="instore-payment-amount-header" />
</Grid >
<Grid item xs=12>
<KeypadEntry className="instore-payment-amount-keypad" />
</Grid >
</Grid >
<Grid item xs=12 md=6 className="instore-payment-types-section">
<Griditem xs=12>
<Typography className="instore-payment-types-header" />
</Grid >
<Grid item xs=12>
<Grid>
<TenderButton className="instore-payment-types-button-${type}" />
//{type} is cash, credit, paybylink, etc.
</Grid >
</Grid >
</Grid >
</Grid >
Payment types HTML rendered on the UI
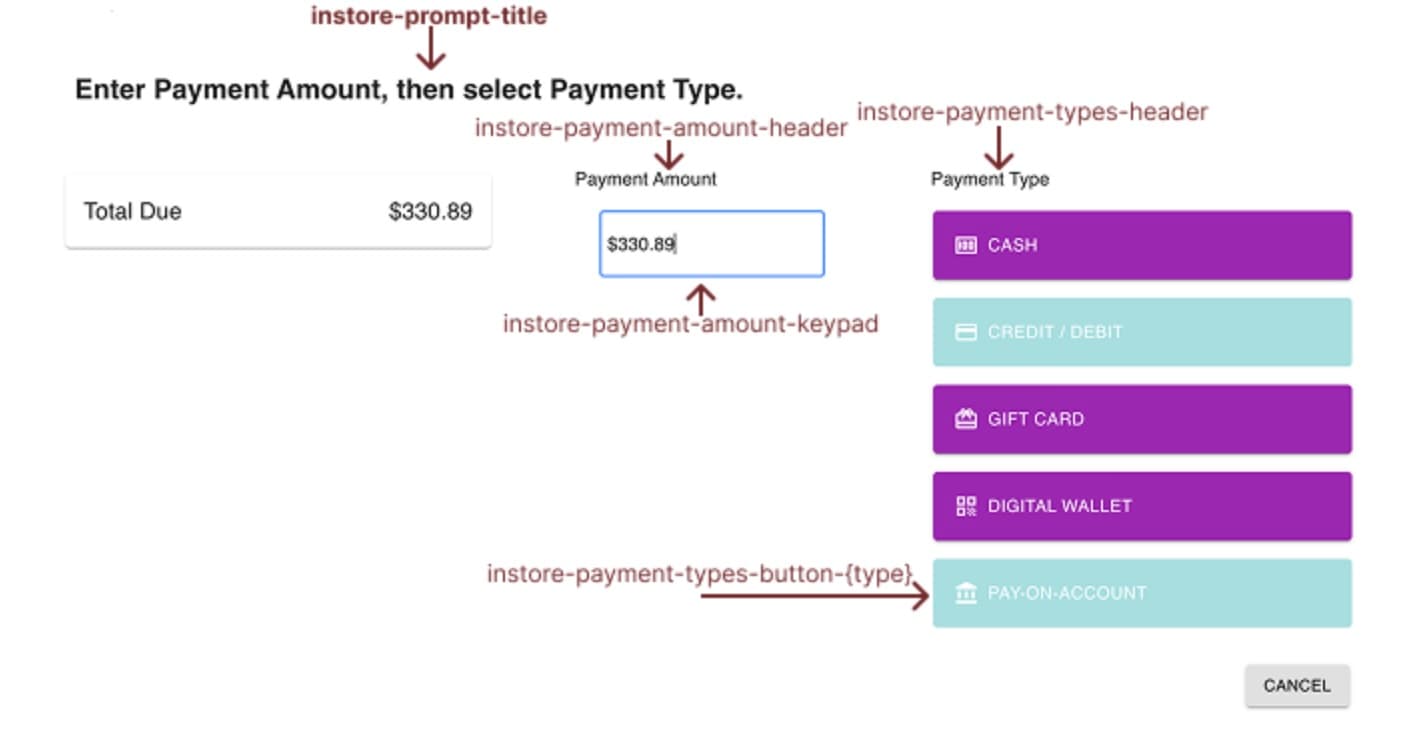
HTML structure for cash
<Grid className="instore-payment-entry-container">
<Grid item xs=12 md=6 className="instore-payment-amount-section">
<Grid item xs=12>
<Typography className="instore-payment-amount-header" />
</Grid >
<Grid item xs=12>
<KeypadEntry className="instore-payment-amount-keypad>" />
</Grid >
</Grid >
<Grid item xs=12 md=6 className="instore-cash-section">
<Grid item xs=12>
<Typography className="instore-cash-header" />
</Grid >
<Grid item xs=12>
<Grid>
<Button className="instore-cash-button-${value}" /> //List of buttons
</Grid>
</Grid>
</Grid>
</Grid>
Cash HTML rendered on the UI
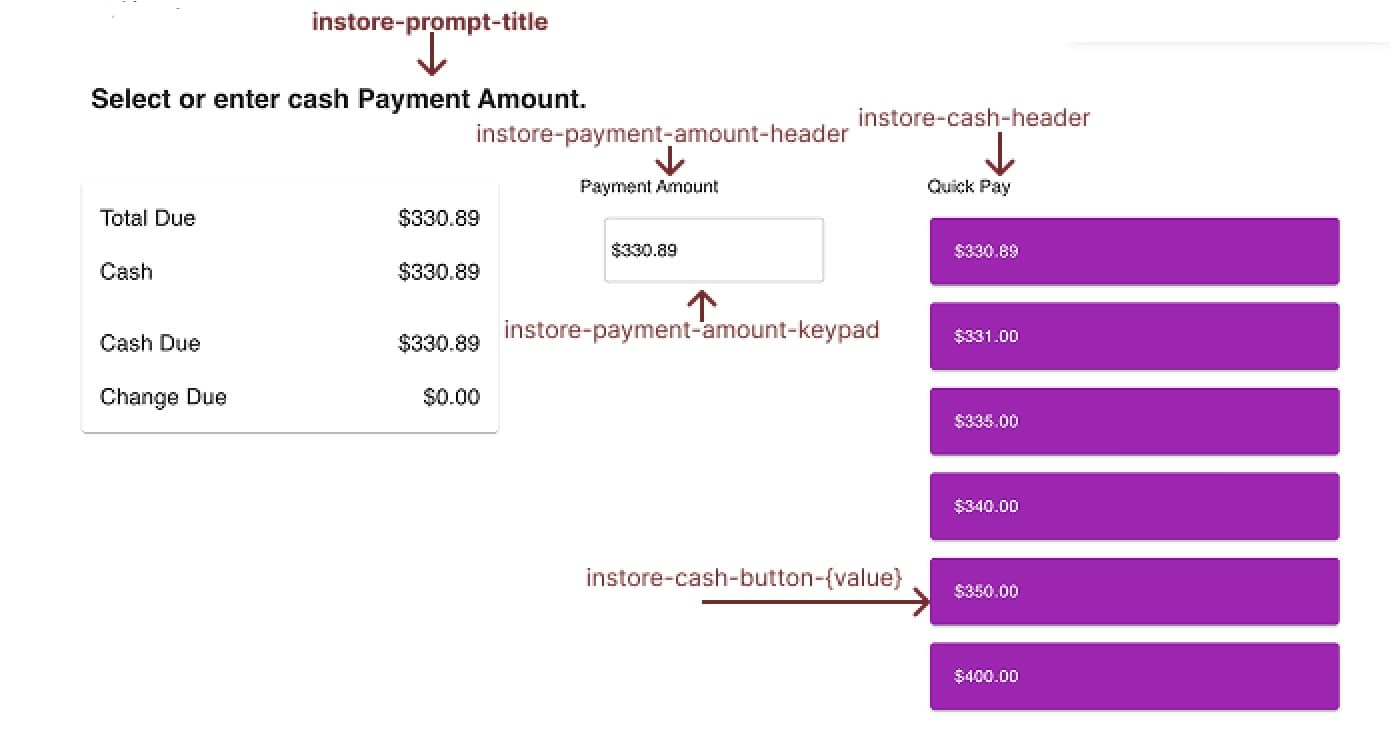
HTML structure for cash drawer
<Grid>
<Grid item xs=12 md=6 >
<Grid item xs=12>
<Typography className="instore-cash-promp-drawer" />
</Grid >
</Grid>
</Grid>
Cash drawer HTML rendered on the UI
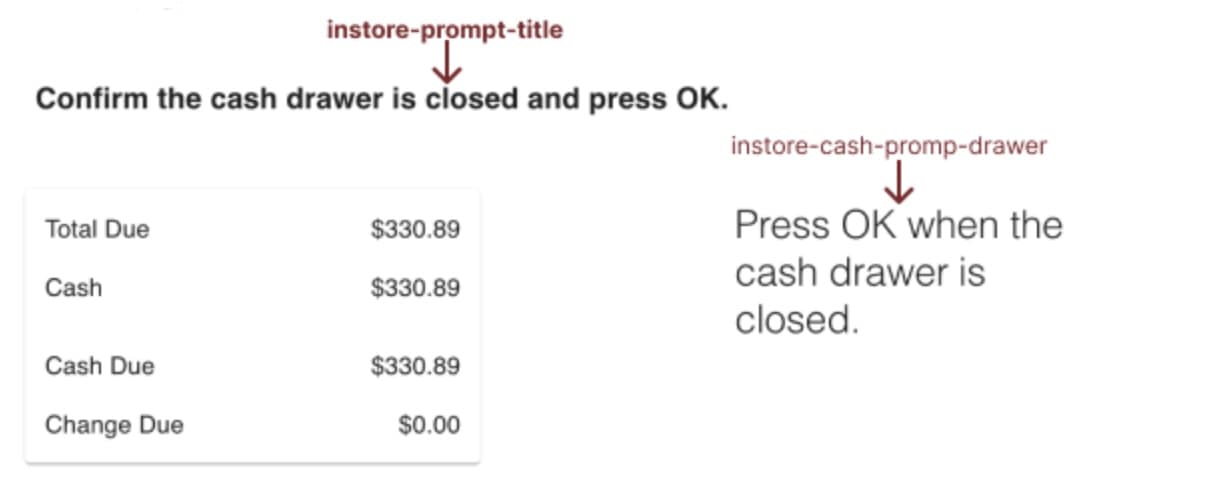
HTML structure for change due
<Grid className="instore-change-due-container">
<Grid item xs=12>
<Typography className="instore-cash-change-row-0" />
<Typography className="instore-cash-change-row-1" />
<Typography className="instore-cash-change-row-2" />
<Typography className="instore-cash-change-row-3" />
</Grid >
</Grid>
Change due HTML rendered on the UI
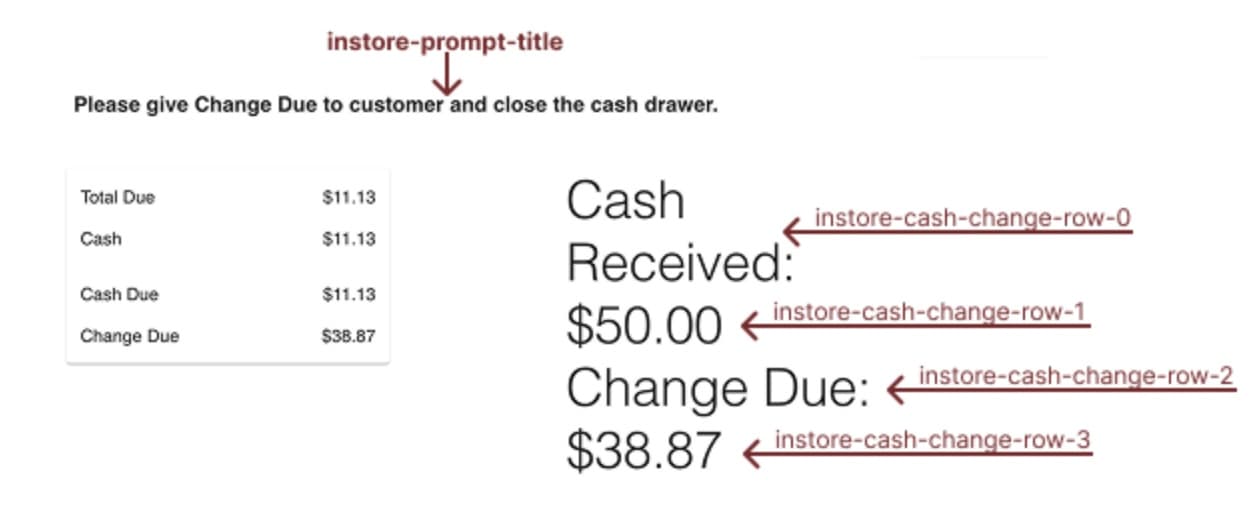
HTML structure for credit processing
<Grid className="instore-payment-entry-container">
<Grid item xs=12>
<Typography className="instore-credit-process-label" />
<Typography className="instore-credit-process-amount" />
</Grid >
</Grid>
Credit processing HTML rendered on the UI
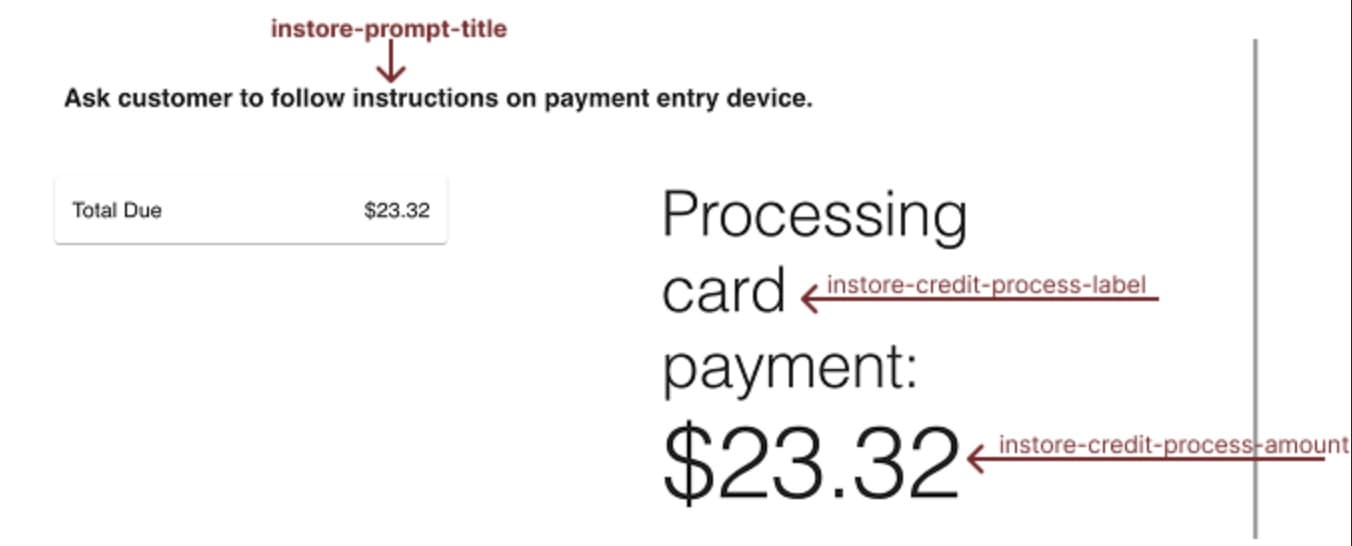
HTML structure for gift card entry
<Grid >
<Grid item xs=12 md=6>
<Grid containerclassName="instore-gift-cart-header">
<Grid item xs=12>
<Button className="instore-gift-cart-camera-button" />
<Typography className="instore-gift-cart-label" />
</Grid >
<Grid item xs=12>
<KeypadEntry className="instore-gift-cart-keypad>" />
</Grid >
</Grid >
</Grid>
</Grid>
Gift card entry HTML rendered on the UI
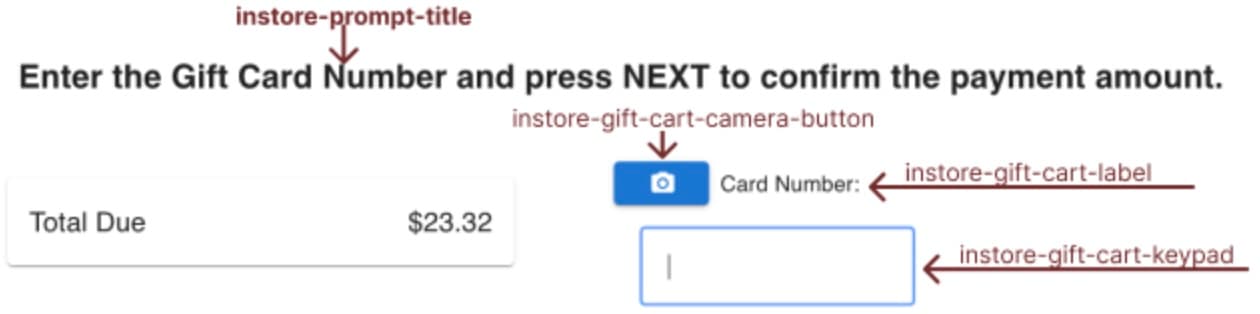
Override strings
By default, InStore retrieves all text snippets from a predefined list of strings. You can display different text by using the InStore API to override this list for the product modules. To override the list, do the following:
- In the InStore GitHub repository, in the client/public folder, locate and copy the
locales
language bundles for product modules. You can use the bundles as a reference when specifying strings to override. - Specify overrides as shown in this example. If you have multiple languages, add a section for each language as shown.
- Define a payload
{ ... }
for each section based on the main payload in the example. The payload only needs to include the strings to be overridden, you don't need to include strings in the payload if you don’t want to override them. - Get an administrator access token. The credentials used here are the same as the ones you use with all InStore APIs.
- Send a PUT request to
/:tenantId/translations
, and then send the payload. You must include{{login_token}}
in the header of your request. For example, in the PUT call, insert the{{login_token}}
as the value for the token key that pairs with the header. - The returned response is the updated bundle in JSON format.
Route
Send the request to the following location:
Path | Type | Description |
---|---|---|
“/:tenantId/translations“ | PUT | Override default translations for supported locales. |
Request
Provide the request in JSON format and include the following fields:
Field | Type | Description |
---|---|---|
translations | object | Configuration for custom translations. |
Example
Cash Drawer
is replaced with Till
for the en-GB
bundle by performing a search and replace in each of the two string files.{
"translations": {
"en-CA": { ... }
"fr-CA": { ... }
"en-GB": {
"App": {
"system_error": "Undefined system error",
"initialization_error": "Unable to initialize session!",
"invalid_request": "Invalid process requested"
},
"PaymentEntry": {
"promptMessage": "Enter Remaining Balance, then select Payment Type.",
"promptMessageAdditionalPayment": "Enter additional remaining balance, then select Payment Type.",
"promptMessageCancel": "Cancelling payment...",
"userMessageCash": "Partial cash tender added",
"buttons": {
"cash": "CASH",
"card": "CREDIT / DEBIT",
"gift": "GIFT CARD",
"wallet": "DIGITAL WALLET"
}
},
"CashCollection": {
"promptMessage": "Select or enter cash Remaining Balance.",
"promptMessageChangeDue": "Please give Change Due to customer and close the till.",
"userMessageCreditApproved": "Card payment approved."
},
"CashDrawer": {
"promptMessageClose": "Confirm the till is closed and press OK.",
"promptMessageSelection": "Select the Till and Reason for the count.",
"promptMessagePayInOpen": "Place cash received in drawer, close the drawer, and press DONE.",
"promptMessagePayOutOpen": "Remove disbursement amount from drawer, close the drawer, and press DONE.",
"promptMessageCashAuditOpen": "Remove transfer amount from drawer, close the drawer, and press DONE.",
"userMessageOpenCashDrawer": "Till open.",
"userMessageCashDrawerClosed": "Till closed."
},
"CashManagement": {
"promptMessageMenu": "Please Select a cash management process.",
"promptMessagePayIn": "Enter the Amount Received and select a Reason.",
"promptMessagePayOut": "Enter the disbursement amount and select a reason.",
"promptMessageCashCount": "Enter the Count for each currency denomination.",
"promptMessageCashCountSummary": "Enter the total counted cash.",
"promptMessageCountAcceptance": "Choose to ACCEPT Count or RECOUNT.",
"promptMessageCashAudit": "Select a Till, review the data and press DONE to finish.",
"promptMessageSafeDeposit": "Select the Till and Reason and press NEXT to continue.",
"promptMessageSafeReserve": "Leave the Reserve Amount in the drawer. Close the drawer. Place the Deposit Amount in the safe, and press DONE.",
"promptMessageBankDepositReserve": "Leave the Reserve Amount in the safe. Send the deposit amount to the bank, and press DONE."
},
...
Override the tender labels
To override the labels for tenders, do the following:
- Log in to the InStore
api-server
by using administrator credentials and retrieve the administrative token. - Enter the token in the header of the request.
- Send a
PUT
request to"{{localhost_url}}/:tenantId/translations"
. - A successful response returns a
202
status code and the updated tender labels in JSON format.
Route
Send the request to the following location:
Path | Type | Description |
---|---|---|
“/:tenantId/translations“ | PUT | Override default translations for supported locales. |
Request
Provide the request in JSON format and include the following fields:
Field | Attribute | Type | Description |
---|---|---|---|
translations | object | Configuration for custom translations. |
Example:
{
"translations": {
"en-US": {
"Checkout": {
"buttons": {
"cash": "CASH",
"typeCards": "CREDIT / DEBIT",
"giftCard": "GIFT CARD",
"digitalWallet": "DIGITAL WALLET",
"poa": "PAY-ON-ACCOUNT",
"payByLink": "PAY BY LINK"
}
}
}
}
}
Override the tender order and icons
To override the tender order and icons, do the following:
- Log in to the InStore
api-server
by using administrator credentials and retrieve the administrative token. - Enter the token in the header of the request.
- Send a
PUT
request to"{{localhost_url}}/:tenantId/payment-buttons"
. - A successful response returns a
202
status code and the updated order and labels in JSON format.
Route
Send the request to the following location:
Path | Type | Description |
---|---|---|
“/:tenantId/payment-buttons“ | PUT | Update the tender display configuration and return the updated tenant document. |
Request
Provide the request in JSON format and include the following fields:
Field | Attribute | Type | Description | Example |
---|---|---|---|---|
paymentButtons | object array | Configuration to set for the tender display. | See below | |
key | string | Key that identifies the payment button to update. | “creditCard” | |
icon | string | Icon to display from MaterialUI icons. | “CreditCard“ | |
order | number(int) | Order in which the payment button will appear (from 1 to 10). | 1 |
Example:
{
"paymentButtons": [
{
"key": "creditCard",
"icon": "CreditCard",
"order": 1
},
{
"key": "cash",
"icon": "Money1",
"order": 2
}
]
}
Override print options
modular_store
. For example, you can omit a receipt option on the cart if certain conditions on the customer record are not met.To override print options, do the following:
-
Import
InStore_State/store
.import `{ useStore }` from "InStore_State/store";
-
Extract
configuration store
in your component fromuseStore
.const { configuration } = useStore();
-
Call
setOverridePrintOptions
in any part of the component to override print options on Checkout or Refund in the InStore_Core module.configuration.setOverridePrintOptions(['Paper']); //Options: Paper, Email, Text, and None
setOverridePrintOptions
is either an array of strings or null
. If this override consists of null
, then InStore uses the values that were set by the Receipt_Print_Options administration parameter in the InStore Center.
An array will replace the values that were configured by the administration parameter.